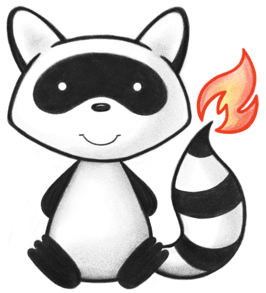
001/*- 002 * #%L 003 * HAPI FHIR Subscription Server 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.topic; 021 022import ca.uhn.fhir.interceptor.model.RequestPartitionId; 023import ca.uhn.fhir.jpa.searchparam.matcher.InMemoryMatchResult; 024import ca.uhn.fhir.jpa.topic.filter.ISubscriptionTopicFilterMatcher; 025import ca.uhn.fhir.rest.api.RestOperationTypeEnum; 026import jakarta.annotation.Nonnull; 027import jakarta.annotation.Nullable; 028import org.hl7.fhir.instance.model.api.IBaseResource; 029 030import java.util.List; 031 032public class SubscriptionTopicDispatchRequest { 033 @Nonnull 034 private final String myTopicUrl; 035 036 @Nonnull 037 private final List<IBaseResource> myResources; 038 039 @Nonnull 040 private final ISubscriptionTopicFilterMatcher mySubscriptionTopicFilterMatcher; 041 042 @Nonnull 043 private final RestOperationTypeEnum myRequestType; 044 045 @Nullable 046 private final InMemoryMatchResult myInMemoryMatchResult; 047 048 @Nullable 049 private final RequestPartitionId myRequestPartitionId; 050 051 @Nullable 052 private final String myTransactionId; 053 054 /** 055 * @param theTopicUrl Deliver to subscriptions for this topic 056 * @param theResources The list of resources to deliver. The first resource will be the primary "focus" resource per the Subscription documentation. 057 * This list should _not_ include the SubscriptionStatus. The SubscriptionStatus will be added as the first element to 058 * the delivered bundle. The reason for this is that the SubscriptionStatus needs to reference the subscription ID, which is 059 * not known until the bundle is delivered. 060 * @param theSubscriptionTopicFilterMatcher is used to match the primary "focus" resource against the subscription filters 061 * @param theRequestType The type of request that led to this dispatch. This determines the request type of the bundle entries 062 * @param theInMemoryMatchResult Information about the match event that led to this dispatch that is sent to SUBSCRIPTION_RESOURCE_MATCHED 063 * @param theRequestPartitionId The request partitions of the request, if any. This is used by subscriptions that need to perform repository 064 * operations as a part of their delivery. Those repository operations will be performed on the supplied request partitions 065 * @param theTransactionId The transaction ID of the request, if any. This is used for logging. 066 * 067 */ 068 public SubscriptionTopicDispatchRequest( 069 @Nonnull String theTopicUrl, 070 @Nonnull List<IBaseResource> theResources, 071 @Nonnull ISubscriptionTopicFilterMatcher theSubscriptionTopicFilterMatcher, 072 @Nonnull RestOperationTypeEnum theRequestType, 073 @Nullable InMemoryMatchResult theInMemoryMatchResult, 074 @Nullable RequestPartitionId theRequestPartitionId, 075 @Nullable String theTransactionId) { 076 myTopicUrl = theTopicUrl; 077 myResources = theResources; 078 mySubscriptionTopicFilterMatcher = theSubscriptionTopicFilterMatcher; 079 myRequestType = theRequestType; 080 myInMemoryMatchResult = theInMemoryMatchResult; 081 myRequestPartitionId = theRequestPartitionId; 082 myTransactionId = theTransactionId; 083 } 084 085 public String getTopicUrl() { 086 return myTopicUrl; 087 } 088 089 public List<IBaseResource> getResources() { 090 return myResources; 091 } 092 093 public ISubscriptionTopicFilterMatcher getSubscriptionTopicFilterMatcher() { 094 return mySubscriptionTopicFilterMatcher; 095 } 096 097 public RestOperationTypeEnum getRequestType() { 098 return myRequestType; 099 } 100 101 public InMemoryMatchResult getInMemoryMatchResult() { 102 return myInMemoryMatchResult; 103 } 104 105 public RequestPartitionId getRequestPartitionId() { 106 return myRequestPartitionId; 107 } 108 109 public String getTransactionId() { 110 return myTransactionId; 111 } 112}