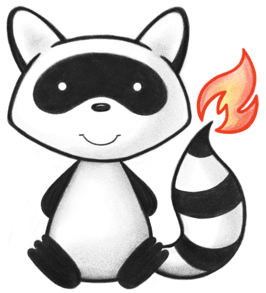
001/*- 002 * #%L 003 * HAPI FHIR Subscription Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.topic; 021 022import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 023import ca.uhn.fhir.rest.param.TokenParam; 024import ca.uhn.fhir.rest.server.util.ISearchParamRegistry; 025import ca.uhn.fhir.subscription.SubscriptionConstants; 026import ca.uhn.hapi.converters.canonical.VersionCanonicalizer; 027import jakarta.annotation.Nonnull; 028import org.hl7.fhir.r5.model.Enumerations; 029import org.hl7.fhir.r5.model.SubscriptionTopic; 030 031public class SubscriptionTopicLoader extends BaseSubscriptionTopicLoader { 032 033 /** 034 * Constructor 035 */ 036 public SubscriptionTopicLoader( 037 VersionCanonicalizer theVersionCanonicalizer, 038 SubscriptionTopicRegistry theSubscriptionTopicRegistry, 039 ISearchParamRegistry theSearchParamRegistry) { 040 super(theVersionCanonicalizer, "SubscriptionTopic", theSubscriptionTopicRegistry, theSearchParamRegistry); 041 } 042 043 @Override 044 @Nonnull 045 public SearchParameterMap getSearchParameterMap() { 046 SearchParameterMap map = new SearchParameterMap(); 047 048 if (mySearchParamRegistry.getActiveSearchParam( 049 "SubscriptionTopic", "status", ISearchParamRegistry.SearchParamLookupContextEnum.ALL) 050 != null) { 051 map.add(SubscriptionTopic.SP_STATUS, new TokenParam(null, Enumerations.PublicationStatus.ACTIVE.toCode())); 052 } 053 map.setLoadSynchronousUpTo(SubscriptionConstants.MAX_SUBSCRIPTION_RESULTS); 054 return map; 055 } 056}