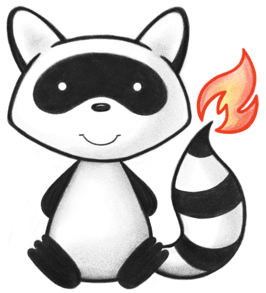
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.api; 021 022import ca.uhn.fhir.mdm.api.paging.MdmPageRequest; 023import ca.uhn.fhir.mdm.api.params.MdmHistorySearchParameters; 024import ca.uhn.fhir.mdm.api.params.MdmQuerySearchParameters; 025import ca.uhn.fhir.mdm.model.MdmCreateOrUpdateParams; 026import ca.uhn.fhir.mdm.model.MdmMergeGoldenResourcesParams; 027import ca.uhn.fhir.mdm.model.MdmTransactionContext; 028import ca.uhn.fhir.mdm.model.MdmUnduplicateGoldenResourceParams; 029import ca.uhn.fhir.mdm.model.mdmevents.MdmLinkJson; 030import ca.uhn.fhir.mdm.model.mdmevents.MdmLinkWithRevisionJson; 031import ca.uhn.fhir.rest.api.server.RequestDetails; 032import ca.uhn.fhir.rest.server.servlet.ServletRequestDetails; 033import jakarta.annotation.Nullable; 034import org.hl7.fhir.instance.model.api.IAnyResource; 035import org.hl7.fhir.instance.model.api.IBaseParameters; 036import org.hl7.fhir.instance.model.api.IPrimitiveType; 037import org.springframework.data.domain.Page; 038 039import java.math.BigDecimal; 040import java.util.List; 041 042public interface IMdmControllerSvc { 043 @Deprecated 044 Page<MdmLinkJson> queryLinks( 045 @Nullable String theGoldenResourceId, 046 @Nullable String theSourceResourceId, 047 @Nullable String theMatchResult, 048 @Nullable String theLinkSource, 049 MdmTransactionContext theMdmTransactionContext, 050 MdmPageRequest thePageRequest); 051 052 @Deprecated 053 Page<MdmLinkJson> queryLinks( 054 @Nullable String theGoldenResourceId, 055 @Nullable String theSourceResourceId, 056 @Nullable String theMatchResult, 057 @Nullable String theLinkSource, 058 MdmTransactionContext theMdmTransactionContext, 059 MdmPageRequest thePageRequest, 060 RequestDetails theRequestDetails); 061 062 Page<MdmLinkJson> queryLinks( 063 MdmQuerySearchParameters theMdmQuerySearchParameters, 064 MdmTransactionContext theMdmTransactionContext, 065 RequestDetails theRequestDetails); 066 067 @Deprecated 068 Page<MdmLinkJson> queryLinksFromPartitionList( 069 @Nullable String theGoldenResourceId, 070 @Nullable String theSourceResourceId, 071 @Nullable String theMatchResult, 072 @Nullable String theLinkSource, 073 MdmTransactionContext theMdmTransactionContext, 074 MdmPageRequest thePageRequest, 075 List<Integer> thePartitionIds); 076 077 Page<MdmLinkJson> queryLinksFromPartitionList( 078 MdmQuerySearchParameters theMdmQuerySearchParameters, MdmTransactionContext theMdmTransactionContext); 079 080 List<MdmLinkWithRevisionJson> queryLinkHistory( 081 MdmHistorySearchParameters theMdmHistorySearchParameters, RequestDetails theRequestDetails); 082 083 Page<MdmLinkJson> getDuplicateGoldenResources( 084 MdmTransactionContext theMdmTransactionContext, MdmPageRequest thePageRequest); 085 086 Page<MdmLinkJson> getDuplicateGoldenResources( 087 MdmTransactionContext theMdmTransactionContext, 088 MdmPageRequest thePageRequest, 089 RequestDetails theRequestDetails, 090 String theRequestResourceType); 091 092 @Deprecated(forRemoval = true, since = "6.8.0") 093 void notDuplicateGoldenResource( 094 String theGoldenResourceId, 095 String theTargetGoldenResourceId, 096 MdmTransactionContext theMdmTransactionContext); 097 098 default void unduplicateGoldenResource(MdmUnduplicateGoldenResourceParams theParams) { 099 notDuplicateGoldenResource( 100 theParams.getGoldenResourceId(), theParams.getTargetGoldenResourceId(), theParams.getMdmContext()); 101 } 102 103 @Deprecated(forRemoval = true, since = "6.8.0") 104 IAnyResource mergeGoldenResources( 105 String theFromGoldenResourceId, 106 String theToGoldenResourceId, 107 IAnyResource theManuallyMergedGoldenResource, 108 MdmTransactionContext theMdmTransactionContext); 109 110 default IAnyResource mergeGoldenResources(MdmMergeGoldenResourcesParams theParams) { 111 return mergeGoldenResources( 112 theParams.getFromGoldenResourceId(), 113 theParams.getToGoldenResourceId(), 114 theParams.getManuallyMergedResource(), 115 theParams.getMdmTransactionContext()); 116 } 117 118 @Deprecated(forRemoval = true, since = "6.8.0") 119 IAnyResource updateLink( 120 String theGoldenResourceId, 121 String theSourceResourceId, 122 String theMatchResult, 123 MdmTransactionContext theMdmTransactionContext); 124 125 default IAnyResource updateLink(MdmCreateOrUpdateParams theParams) { 126 String matchResult = theParams.getMatchResult() == null 127 ? null 128 : theParams.getMatchResult().name(); 129 return updateLink( 130 theParams.getGoldenResourceId(), theParams.getResourceId(), matchResult, theParams.getMdmContext()); 131 } 132 133 @Deprecated(forRemoval = true, since = "6.8.0") 134 IAnyResource createLink( 135 String theGoldenResourceId, 136 String theSourceResourceId, 137 @Nullable String theMatchResult, 138 MdmTransactionContext theMdmTransactionContext); 139 140 default IAnyResource createLink(MdmCreateOrUpdateParams theParams) { 141 String matchResult = theParams.getMatchResult() == null 142 ? null 143 : theParams.getMatchResult().name(); 144 return createLink( 145 theParams.getGoldenResourceId(), theParams.getResourceId(), matchResult, theParams.getMdmContext()); 146 } 147 148 IBaseParameters submitMdmClearJob( 149 List<String> theResourceNames, 150 IPrimitiveType<BigDecimal> theBatchSize, 151 ServletRequestDetails theRequestDetails); 152 153 IBaseParameters submitMdmSubmitJob( 154 List<String> theUrls, IPrimitiveType<BigDecimal> theBatchSize, ServletRequestDetails theRequestDetails); 155}