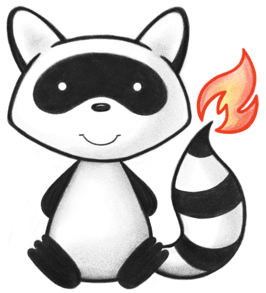
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.api; 021 022import ca.uhn.fhir.jpa.model.entity.PartitionablePartitionId; 023import ca.uhn.fhir.rest.api.server.storage.IResourcePersistentId; 024 025import java.util.Date; 026 027public interface IMdmLink<T extends IResourcePersistentId<?>> { 028 T getId(); 029 030 IMdmLink<T> setId(T theId); 031 032 T getGoldenResourcePersistenceId(); 033 034 IMdmLink<T> setGoldenResourcePersistenceId(T theGoldenResourcePid); 035 036 T getSourcePersistenceId(); 037 038 IMdmLink<T> setSourcePersistenceId(T theSourcePid); 039 040 MdmMatchResultEnum getMatchResult(); 041 042 IMdmLink<T> setMatchResult(MdmMatchResultEnum theMatchResult); 043 044 default boolean isNoMatch() { 045 return getMatchResult() == MdmMatchResultEnum.NO_MATCH; 046 } 047 048 default boolean isMatch() { 049 return getMatchResult() == MdmMatchResultEnum.MATCH; 050 } 051 052 default boolean isPossibleMatch() { 053 return getMatchResult() == MdmMatchResultEnum.POSSIBLE_MATCH; 054 } 055 056 default boolean isRedirect() { 057 return getMatchResult() == MdmMatchResultEnum.REDIRECT; 058 } 059 060 default boolean isPossibleDuplicate() { 061 return getMatchResult() == MdmMatchResultEnum.POSSIBLE_DUPLICATE; 062 } 063 064 MdmLinkSourceEnum getLinkSource(); 065 066 IMdmLink<T> setLinkSource(MdmLinkSourceEnum theLinkSource); 067 068 default boolean isAuto() { 069 return getLinkSource() == MdmLinkSourceEnum.AUTO; 070 } 071 072 default boolean isManual() { 073 return getLinkSource() == MdmLinkSourceEnum.MANUAL; 074 } 075 076 Date getCreated(); 077 078 IMdmLink<T> setCreated(Date theCreated); 079 080 Date getUpdated(); 081 082 IMdmLink<T> setUpdated(Date theUpdated); 083 084 String getVersion(); 085 086 IMdmLink<T> setVersion(String theVersion); 087 088 Boolean getEidMatch(); 089 090 Boolean isEidMatchPresent(); 091 092 IMdmLink<T> setEidMatch(Boolean theEidMatch); 093 094 Boolean getHadToCreateNewGoldenResource(); 095 096 IMdmLink<T> setHadToCreateNewGoldenResource(Boolean theHadToCreateNewGoldenResource); 097 098 Long getVector(); 099 100 IMdmLink<T> setVector(Long theVector); 101 102 Double getScore(); 103 104 IMdmLink<T> setScore(Double theScore); 105 106 Long getRuleCount(); 107 108 IMdmLink<T> setRuleCount(Long theRuleCount); 109 110 String getMdmSourceType(); 111 112 IMdmLink<T> setMdmSourceType(String theMdmSourceType); 113 114 void setPartitionId(PartitionablePartitionId thePartitionablePartitionId); 115 116 PartitionablePartitionId getPartitionId(); 117}