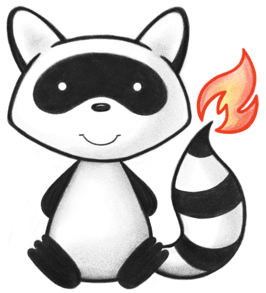
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.api; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.mdm.rules.json.MdmRulesJson; 024 025import java.util.stream.Collectors; 026 027public interface IMdmSettings { 028 029 String EMPI_CHANNEL_NAME = "empi"; 030 031 // Parallel processing of MDM can result in missed matches. Best to single-thread. 032 int MDM_DEFAULT_CONCURRENT_CONSUMERS = 1; 033 034 boolean isEnabled(); 035 036 int getConcurrentConsumers(); 037 038 MdmRulesJson getMdmRules(); 039 040 boolean isPreventEidUpdates(); 041 042 boolean isPreventMultipleEids(); 043 044 String getRuleVersion(); 045 046 String getSurvivorshipRules(); 047 048 default boolean isSupportedMdmType(String theResourceName) { 049 return getMdmRules().getMdmTypes().contains(theResourceName); 050 } 051 052 default String getSupportedMdmTypes() { 053 return getMdmRules().getMdmTypes().stream().collect(Collectors.joining(", ")); 054 } 055 056 int getCandidateSearchLimit(); 057 058 String getGoldenResourcePartitionName(); 059 060 void setGoldenResourcePartitionName(String theGoldenResourcePartitionName); 061 062 boolean getSearchAllPartitionForMatch(); 063 064 void setSearchAllPartitionForMatch(boolean theSearchAllPartitionForMatch); 065 066 // TODO: on next bump, make this method non-default 067 default boolean isAutoExpungeGoldenResources() { 068 return false; 069 } 070 071 // TODO: on next bump, make this method non-default 072 default void setAutoExpungeGoldenResources(boolean theShouldAutoExpunge) { 073 throw new UnsupportedOperationException(Msg.code(2427)); 074 } 075 076 // In MATCH_ONLY mode, the Patient/$match operation is available, but no mdm processing takes place. 077 default MdmModeEnum getMode() { 078 return MdmModeEnum.MATCH_AND_LINK; 079 } 080}