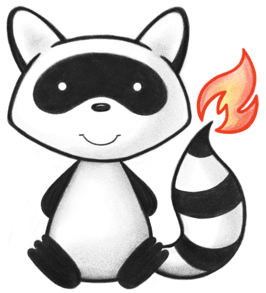
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.api; 021 022import ca.uhn.fhir.rest.api.server.RequestDetails; 023import jakarta.annotation.Nullable; 024import org.hl7.fhir.instance.model.api.IIdType; 025 026public interface IMdmSubmitSvc { 027 028 /** 029 * Submit all eligible resources for MDM processing. 030 * 031 * @param theCriteria The FHIR search critieria for filtering the resources to be submitted for MDM processing. 032 * NOTE: 033 * When using this function, the criteria supplied must be valid for all MDM types. e.g. , if you 034 * run this with the criteria birthDate=1990-06-28, it will fail, as Practitioners do not have a birthday. 035 * Use with caution. 036 * 037 * @return 038 */ 039 long submitAllSourceTypesToMdm(@Nullable String theCriteria, RequestDetails theRequestDetails); 040 041 /** 042 * Given a type and a search criteria, submit all found resources for MDM processing. 043 * 044 * @param theSourceResourceType the resource type that you wish to execute a search over for submission to MDM. 045 * @param theCriteria The FHIR search critieria for filtering the resources to be submitted for MDM processing.. 046 * @return the number of resources submitted for MDM processing. 047 */ 048 long submitSourceResourceTypeToMdm( 049 String theSourceResourceType, String theCriteria, RequestDetails theRequestDetails); 050 051 /** 052 * Convenience method that calls {@link #submitSourceResourceTypeToMdm(String, String)} with the type pre-populated. 053 * 054 * @param theCriteria The FHIR search critieria for filtering the resources to be submitted for MDM processing. 055 * @return the number of resources submitted for MDM processing. 056 */ 057 @Deprecated(forRemoval = true, since = "6.8.0") 058 long submitPractitionerTypeToMdm(String theCriteria, RequestDetails theRequestDetails); 059 060 /** 061 * Convenience method that calls {@link #submitSourceResourceTypeToMdm(String, String)} with the type pre-populated. 062 * 063 * @param theCriteria The FHIR search critieria for filtering the resources to be submitted for MDM processing. 064 * @return the number of resources submitted for MDM processing. 065 */ 066 @Deprecated(forRemoval = true, since = "6.8.0") 067 long submitPatientTypeToMdm(String theCriteria, RequestDetails theRequestDetails); 068 069 /** 070 * Given an ID and a source resource type valid for MDM, manually submit the given ID for MDM processing. 071 * 072 * @param theId the ID of the resource to process for MDM. 073 * @return the constant `1`, as if this function returns successfully, it will have processed one resource for MDM. 074 */ 075 long submitSourceResourceToMdm(IIdType theId, RequestDetails theRequestDetails); 076 077 /** 078 * This setter exists to allow imported modules to override settings. 079 * 080 * @param theMdmSettings Settings to set 081 */ 082 void setMdmSettings(IMdmSettings theMdmSettings); 083 084 /** 085 * Buffer size for fetching results to add to MDM queue. 086 * 087 * @param theBufferSize 088 */ 089 public void setBufferSize(int theBufferSize); 090}