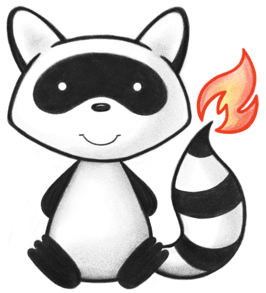
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.api; 021 022import ca.uhn.fhir.mdm.model.MdmTransactionContext; 023import ca.uhn.fhir.rest.api.server.RequestDetails; 024import org.hl7.fhir.instance.model.api.IBase; 025 026/** 027 * Service that applies survivorship rules on target and golden resources. 028 */ 029public interface IMdmSurvivorshipService { 030 031 /** 032 * Merges two golden resources by overwriting all field values on theGoldenResource param for CREATE_RESOURCE, 033 * UPDATE_RESOURCE, SUBMIT_RESOURCE_TO_MDM, UPDATE_LINK (when setting to MATCH) and MANUAL_MERGE_GOLDEN_RESOURCES. 034 * PID, identifiers and meta values are not affected by this operation. 035 * 036 * Applies survivorship rules to merge fields from the specified target resource to the golden resource. Survivorship 037 * rules may include, but not limited to the following data consolidation methods: 038 * 039 * <ul> 040 * <li> 041 * Length of field - apply the field value containing most or least number of characters - e.g. longest name 042 * </li> 043 * <li> 044 * Date time - all the field value from the oldest or the newest recrod - e.g. use the most recent phone number 045 * </li> 046 * <li> 047 * Frequency - use the most or least frequent number of occurrence - e.g. most common phone number 048 * </li> 049 * <li> 050 * Integer - number functions (largest, sum, avg) - e.g. number of patient encounters 051 * </li> 052 * <li> 053 * Quality of data - best quality data - e.g. data coming from a certain system is considered trusted and overrides 054 * all other values 055 * </li> 056 * <li> 057 * A hybrid approach combining all methods listed above as best fits 058 * </li> 059 * </ul> 060 * 061 * @param theTargetResource Target resource to merge fields from 062 * @param theGoldenResource Golden resource to merge fields into 063 * @param theMdmTransactionContext Current transaction context 064 * @param <T> Resource type to apply the survivorship rules to 065 */ 066 <T extends IBase> void applySurvivorshipRulesToGoldenResource( 067 T theTargetResource, T theGoldenResource, MdmTransactionContext theMdmTransactionContext); 068 069 /** 070 * GoldenResources can have non-empty field data created from changes to the various 071 * resources that are matched to it (using some pre-defined survivorship rules). 072 * 073 * If a match link between a source and golden resource is broken, this method 074 * will rebuild/repopulate the GoldenResource based on the current links 075 * and current survivorship rules. 076 * 077 * @param theGoldenResource - the golden resource to rebuild 078 * @param theMdmTransactionContext - the transaction context 079 * @param <T> - Resource type to apply the survivorship rules to 080 */ 081 <T extends IBase> T rebuildGoldenResourceWithSurvivorshipRules( 082 RequestDetails theRequestDetails, T theGoldenResource, MdmTransactionContext theMdmTransactionContext); 083}