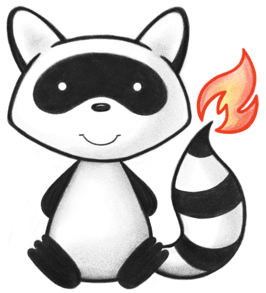
001package ca.uhn.fhir.mdm.api; 002 003/*- 004 * #%L 005 * HAPI FHIR - Master Data Management 006 * %% 007 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 008 * %% 009 * Licensed under the Apache License, Version 2.0 (the "License"); 010 * you may not use this file except in compliance with the License. 011 * You may obtain a copy of the License at 012 * 013 * http://www.apache.org/licenses/LICENSE-2.0 014 * 015 * Unless required by applicable law or agreed to in writing, software 016 * distributed under the License is distributed on an "AS IS" BASIS, 017 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 018 * See the License for the specific language governing permissions and 019 * limitations under the License. 020 * #L% 021 */ 022 023import ca.uhn.fhir.jpa.model.entity.EnversRevision; 024import org.apache.commons.lang3.builder.ToStringBuilder; 025 026import java.util.Objects; 027 028public class MdmLinkWithRevision<T extends IMdmLink<?>> { 029 private final T myMdmLink; 030 private final EnversRevision myEnversRevision; 031 032 public MdmLinkWithRevision(T theMdmLink, EnversRevision theEnversRevision) { 033 myMdmLink = theMdmLink; 034 myEnversRevision = theEnversRevision; 035 } 036 037 public T getMdmLink() { 038 return myMdmLink; 039 } 040 041 public EnversRevision getEnversRevision() { 042 return myEnversRevision; 043 } 044 045 @Override 046 public boolean equals(Object theO) { 047 if (this == theO) return true; 048 if (theO == null || getClass() != theO.getClass()) return false; 049 final MdmLinkWithRevision<?> that = (MdmLinkWithRevision<?>) theO; 050 return Objects.equals(myMdmLink, that.myMdmLink) && Objects.equals(myEnversRevision, that.myEnversRevision); 051 } 052 053 @Override 054 public int hashCode() { 055 return Objects.hash(myMdmLink, myEnversRevision); 056 } 057 058 @Override 059 public String toString() { 060 return new ToStringBuilder(this) 061 .append("myMdmLink", myMdmLink) 062 .append("myEnversRevision", myEnversRevision) 063 .toString(); 064 } 065}