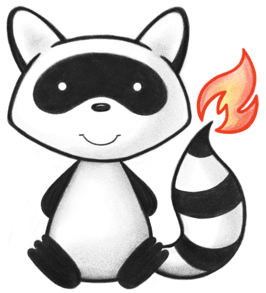
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.dao; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.mdm.api.IMdmLink; 024import ca.uhn.fhir.mdm.api.MdmLinkSourceEnum; 025import ca.uhn.fhir.mdm.api.MdmLinkWithRevision; 026import ca.uhn.fhir.mdm.api.MdmMatchResultEnum; 027import ca.uhn.fhir.mdm.api.paging.MdmPageRequest; 028import ca.uhn.fhir.mdm.api.params.MdmHistorySearchParameters; 029import ca.uhn.fhir.mdm.api.params.MdmQuerySearchParameters; 030import ca.uhn.fhir.mdm.model.MdmPidTuple; 031import ca.uhn.fhir.rest.api.server.storage.IResourcePersistentId; 032import ca.uhn.fhir.rest.server.exceptions.UnprocessableEntityException; 033import org.hl7.fhir.instance.model.api.IIdType; 034import org.springframework.data.domain.Example; 035import org.springframework.data.domain.Page; 036import org.springframework.data.domain.Pageable; 037import org.springframework.data.history.Revisions; 038 039import java.util.Collection; 040import java.util.Date; 041import java.util.List; 042import java.util.Optional; 043 044public interface IMdmLinkDao<P extends IResourcePersistentId<?>, M extends IMdmLink<P>> { 045 int deleteWithAnyReferenceToPid(P thePid); 046 047 int deleteWithAnyReferenceToPidAndMatchResultNot(P thePid, MdmMatchResultEnum theMatchResult); 048 049 List<MdmPidTuple<P>> expandPidsFromGroupPidGivenMatchResult( 050 P theGroupPid, MdmMatchResultEnum theMdmMatchResultEnum); 051 052 List<MdmPidTuple<P>> expandPidsBySourcePidAndMatchResult(P theSourcePid, MdmMatchResultEnum theMdmMatchResultEnum); 053 054 List<MdmPidTuple<P>> expandPidsByGoldenResourcePidAndMatchResult( 055 P theSourcePid, MdmMatchResultEnum theMdmMatchResultEnum); 056 057 // TODO: on next bump, make this method non-default 058 default List<M> findLinksAssociatedWithGoldenResourceOfSourceResourceExcludingNoMatch(P theSourcePid) { 059 throw new UnsupportedOperationException(Msg.code(2428)); 060 } 061 062 List<P> findPidByResourceNameAndThreshold(String theResourceName, Date theHighThreshold, Pageable thePageable); 063 064 List<P> findPidByResourceNameAndThresholdAndPartitionId( 065 String theResourceName, Date theHighThreshold, List<Integer> thePartitionIds, Pageable thePageable); 066 067 List<M> findAllById(List<P> thePids); 068 069 Optional<M> findById(P thePid); 070 071 void deleteAll(List<M> theLinks); 072 073 List<M> findAll(Example<M> theExample); 074 075 List<M> findAll(); 076 077 Long count(); 078 079 void deleteAll(); 080 081 M save(M theMdmLink); 082 083 Optional<M> findOne(Example<M> theExample); 084 085 void delete(M theMdmLink); 086 087 // TODO KHS is this method still required? Probably not? But leaving it in for now... 088 M validateMdmLink(IMdmLink theMdmLink) throws UnprocessableEntityException; 089 090 @Deprecated 091 Page<M> search( 092 IIdType theGoldenResourceId, 093 IIdType theSourceId, 094 MdmMatchResultEnum theMatchResult, 095 MdmLinkSourceEnum theLinkSource, 096 MdmPageRequest thePageRequest, 097 List<Integer> thePartitionId); 098 099 Page<M> search(MdmQuerySearchParameters theMdmQuerySearchParameters); 100 101 Optional<M> findBySourcePidAndMatchResult(P theSourcePid, MdmMatchResultEnum theMatch); 102 103 void deleteLinksWithAnyReferenceToPids(List<P> theResourcePersistentIds); 104 105 // TODO: LD: delete for good on the next bump 106 @Deprecated(since = "6.5.6", forRemoval = true) 107 default Revisions<Long, M> findHistory(P thePid) { 108 throw new UnsupportedOperationException(Msg.code(2296) + "Deprecated and not supported in non-JPA"); 109 } 110 111 default List<MdmLinkWithRevision<M>> getHistoryForIds(MdmHistorySearchParameters theMdmHistorySearchParameters) { 112 throw new UnsupportedOperationException(Msg.code(2299) + "not yet implemented"); 113 } 114 115 /** 116 * Given a collection of PIDs, resolves the associated golden resource IDs. If any of the PIDs 117 * are golden resources, the associated non-golden resources are also fetched. 118 */ 119 default Collection<MdmPidTuple<P>> resolveGoldenResources(List<P> theSourcePids) { 120 throw new UnsupportedOperationException(Msg.code(2568) + "not yet implemented"); 121 } 122}