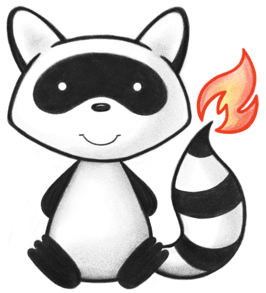
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.dao; 021 022import ca.uhn.fhir.mdm.api.IMdmLink; 023import ca.uhn.fhir.mdm.api.IMdmSettings; 024import org.springframework.beans.factory.annotation.Autowired; 025 026/** 027 * Creates a new {@link IMdmLink} either with the current {@link IMdmSettings#getRuleVersion()} or with a null version. 028 * <br> 029 * **Use extreme caution**. The recommended practice is to only use (@link #newMdmLink()} when WRITING an MDM record. 030 * <br> 031 * Otherwise, there is the risk that code that searches for an MDM record fill fail to locate it due to a version mismatch. 032 * <br> 033 * Database code makes use of SpringData {@link org.springframework.data.domain.Example} queries. 034 */ 035public class MdmLinkFactory<M extends IMdmLink> { 036 private final IMdmSettings myMdmSettings; 037 private final IMdmLinkImplFactory<M> myMdmLinkImplFactory; 038 039 @Autowired 040 public MdmLinkFactory(IMdmSettings theMdmSettings, IMdmLinkImplFactory<M> theMdmLinkImplFactory) { 041 myMdmSettings = theMdmSettings; 042 myMdmLinkImplFactory = theMdmLinkImplFactory; 043 } 044 045 /** 046 * Create a new {@link IMdmLink}, populating it with the version of the ruleset used to create it. 047 * 048 * Use this method **only** when writing a new MDM record. 049 * 050 * @return the new {@link IMdmLink} 051 */ 052 public M newMdmLink() { 053 M retval = myMdmLinkImplFactory.newMdmLinkImpl(); 054 retval.setVersion(myMdmSettings.getRuleVersion()); 055 return retval; 056 } 057 058 /** 059 * Creating a new {@link IMdmLink} with the version deliberately omitted. It will return as null. 060 * 061 * This is the recommended use when querying for any MDM records 062 */ 063 public M newMdmLinkVersionless() { 064 return myMdmLinkImplFactory.newMdmLinkImpl(); 065 } 066}