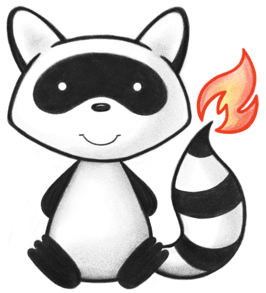
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.interceptor; 021 022import ca.uhn.fhir.interceptor.api.Hook; 023import ca.uhn.fhir.interceptor.api.Interceptor; 024import ca.uhn.fhir.interceptor.api.Pointcut; 025import ca.uhn.fhir.jpa.api.config.JpaStorageSettings; 026import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 027import ca.uhn.fhir.mdm.api.MdmConstants; 028import ca.uhn.fhir.mdm.svc.MdmSearchExpansionSvc; 029import ca.uhn.fhir.rest.api.server.RequestDetails; 030import ca.uhn.fhir.rest.param.ReferenceParam; 031import ca.uhn.fhir.rest.param.TokenParam; 032import ca.uhn.fhir.rest.server.util.ICachedSearchDetails; 033import org.springframework.beans.factory.annotation.Autowired; 034 035/** 036 * This interceptor replaces the auto-generated CapabilityStatement that is generated 037 * by the HAPI FHIR Server with a static hard-coded resource. 038 */ 039@Interceptor 040public class MdmSearchExpandingInterceptor { 041 042 private static final MdmSearchExpansionSvc.IParamTester PARAM_TESTER = (paramName, param) -> { 043 boolean retVal = false; 044 if (param instanceof ReferenceParam) { 045 retVal = ((ReferenceParam) param).isMdmExpand(); 046 } else if (param instanceof TokenParam) { 047 retVal = ((TokenParam) param).isMdmExpand(); 048 } 049 return retVal; 050 }; 051 052 @Autowired 053 private JpaStorageSettings myStorageSettings; 054 055 @Autowired 056 private MdmSearchExpansionSvc myMdmSearchExpansionSvc; 057 058 @Hook( 059 value = Pointcut.STORAGE_PRESEARCH_REGISTERED, 060 order = MdmConstants.ORDER_PRESEARCH_REGISTERED_MDM_SEARCH_EXPANDING_INTERCEPTOR) 061 public void hook( 062 RequestDetails theRequestDetails, 063 SearchParameterMap theSearchParameterMap, 064 ICachedSearchDetails theSearchDetails) { 065 066 if (myStorageSettings.isAllowMdmExpansion()) { 067 String resourceType = theSearchDetails.getResourceType(); 068 myMdmSearchExpansionSvc.expandSearchAndStoreInRequestDetails( 069 resourceType, theRequestDetails, theSearchParameterMap, PARAM_TESTER); 070 } 071 } 072}