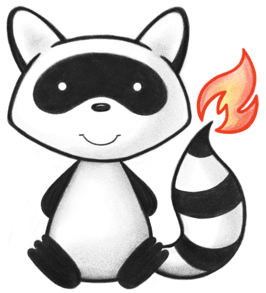
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.model; 021 022import ca.uhn.fhir.mdm.api.MdmMatchResultEnum; 023import ca.uhn.fhir.rest.api.server.RequestDetails; 024import org.hl7.fhir.instance.model.api.IAnyResource; 025 026public class MdmCreateOrUpdateParams { 027 /** 028 * The golden resource id 029 */ 030 private String myGoldenResourceId; 031 032 /** 033 * The golden resource to update for the link. 034 */ 035 private IAnyResource myGoldenResource; 036 037 /** 038 * The source id. 039 */ 040 private String myResourceId; 041 042 /** 043 * The source resource linked to the golden resource 044 */ 045 private IAnyResource mySourceResource; 046 /** 047 * Match result to update the link to. 048 */ 049 private MdmMatchResultEnum myMatchResult; 050 /** 051 * The context. 052 */ 053 private MdmTransactionContext myMdmContext; 054 055 /** 056 * The request details. 057 */ 058 private RequestDetails myRequestDetails; 059 060 public String getGoldenResourceId() { 061 return myGoldenResourceId; 062 } 063 064 public void setGoldenResourceId(String theGoldenResourceId) { 065 myGoldenResourceId = theGoldenResourceId; 066 } 067 068 public String getResourceId() { 069 return myResourceId; 070 } 071 072 public void setResourceId(String theResourceId) { 073 myResourceId = theResourceId; 074 } 075 076 public IAnyResource getGoldenResource() { 077 return myGoldenResource; 078 } 079 080 public void setGoldenResource(IAnyResource theGoldenResource) { 081 myGoldenResource = theGoldenResource; 082 } 083 084 public IAnyResource getSourceResource() { 085 return mySourceResource; 086 } 087 088 public void setSourceResource(IAnyResource theSourceResource) { 089 mySourceResource = theSourceResource; 090 } 091 092 public MdmMatchResultEnum getMatchResult() { 093 return myMatchResult; 094 } 095 096 public void setMatchResult(MdmMatchResultEnum theMatchResult) { 097 myMatchResult = theMatchResult; 098 } 099 100 public MdmTransactionContext getMdmContext() { 101 return myMdmContext; 102 } 103 104 public void setMdmContext(MdmTransactionContext theMdmContext) { 105 myMdmContext = theMdmContext; 106 } 107 108 public RequestDetails getRequestDetails() { 109 return myRequestDetails; 110 } 111 112 public void setRequestDetails(RequestDetails theRequestDetails) { 113 myRequestDetails = theRequestDetails; 114 } 115}