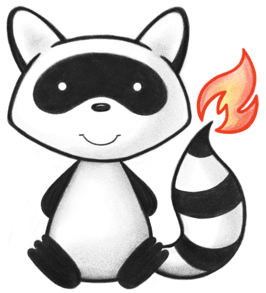
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.model; 021 022import ca.uhn.fhir.mdm.api.MdmLinkSourceEnum; 023import ca.uhn.fhir.mdm.api.MdmMatchResultEnum; 024import ca.uhn.fhir.model.api.IModelJson; 025import com.fasterxml.jackson.annotation.JsonProperty; 026 027import java.util.HashMap; 028import java.util.LinkedHashMap; 029import java.util.Map; 030 031public class MdmMetrics extends MdmResourceMetrics implements IModelJson { 032 033 @JsonProperty("resourceType") 034 private String myResourceType; 035 036 /** 037 * A mapping of MatchType -> LinkSource -> count. 038 * Eg: 039 * MATCH 040 * AUTO - 2 041 * MANUAL - 1 042 * NO_MATCH 043 * AUTO - 1 044 * MANUAL - 3 045 */ 046 @JsonProperty("matchResult2linkSource2count") 047 private Map<MdmMatchResultEnum, Map<MdmLinkSourceEnum, Long>> myMatchTypeToLinkToCountMap; 048 049 /** 050 * Score buckets (in brackets of 0.01 size, and null) to counts. 051 */ 052 @JsonProperty("scoreCounts") 053 private Map<String, Long> myScoreCounts; 054 055 /** 056 * The number of golden resources. 057 */ 058 @JsonProperty("goldenResources") 059 private long myGoldenResourcesCount; 060 061 /** 062 * The number of source resources. 063 */ 064 @JsonProperty("sourceResources") 065 private long mySourceResourcesCount; 066 067 /** 068 * The number of excluded resources. 069 * These are necessarily a subset of both 070 * GoldenResources and SourceResources 071 * (as each Blocked resource will still generate 072 * a GoldenResource) 073 */ 074 @JsonProperty("excludedResources") 075 private long myExcludedResources; 076 077 public String getResourceType() { 078 return myResourceType; 079 } 080 081 public Map<MdmMatchResultEnum, Map<MdmLinkSourceEnum, Long>> getMatchTypeToLinkToCountMap() { 082 if (myMatchTypeToLinkToCountMap == null) { 083 myMatchTypeToLinkToCountMap = new HashMap<>(); 084 } 085 return myMatchTypeToLinkToCountMap; 086 } 087 088 public void addMetric( 089 MdmMatchResultEnum theMdmMatchResultEnum, MdmLinkSourceEnum theLinkSourceEnum, long theCount) { 090 Map<MdmMatchResultEnum, Map<MdmLinkSourceEnum, Long>> map = getMatchTypeToLinkToCountMap(); 091 092 if (!map.containsKey(theMdmMatchResultEnum)) { 093 map.put(theMdmMatchResultEnum, new HashMap<>()); 094 } 095 Map<MdmLinkSourceEnum, Long> lsToCountMap = map.get(theMdmMatchResultEnum); 096 lsToCountMap.put(theLinkSourceEnum, theCount); 097 } 098 099 public void setResourceType(String theResourceType) { 100 myResourceType = theResourceType; 101 } 102 103 public long getGoldenResourcesCount() { 104 return myGoldenResourcesCount; 105 } 106 107 public void setGoldenResourcesCount(long theGoldenResourcesCount) { 108 myGoldenResourcesCount = theGoldenResourcesCount; 109 } 110 111 public long getSourceResourcesCount() { 112 return mySourceResourcesCount; 113 } 114 115 public void setSourceResourcesCount(long theSourceResourcesCount) { 116 mySourceResourcesCount = theSourceResourcesCount; 117 } 118 119 public long getExcludedResources() { 120 return myExcludedResources; 121 } 122 123 public void setExcludedResources(long theExcludedResources) { 124 myExcludedResources = theExcludedResources; 125 } 126 127 public Map<String, Long> getScoreCounts() { 128 if (myScoreCounts == null) { 129 myScoreCounts = new LinkedHashMap<>(); 130 } 131 return myScoreCounts; 132 } 133 134 public void addScore(String theScore, Long theCount) { 135 getScoreCounts().put(theScore, theCount); 136 } 137 138 public static MdmMetrics fromSeperableMetrics( 139 MdmResourceMetrics theMdmResourceMetrics, 140 MdmLinkMetrics theLinkMetrics, 141 MdmLinkScoreMetrics theLinkScoreMetrics) { 142 MdmMetrics metrics = new MdmMetrics(); 143 metrics.setResourceType(theMdmResourceMetrics.getResourceType()); 144 metrics.setExcludedResources(theMdmResourceMetrics.getExcludedResources()); 145 metrics.setGoldenResourcesCount(theMdmResourceMetrics.getGoldenResourcesCount()); 146 metrics.setSourceResourcesCount(theMdmResourceMetrics.getSourceResourcesCount()); 147 metrics.myMatchTypeToLinkToCountMap = theLinkMetrics.getMatchTypeToLinkToCountMap(); 148 metrics.myScoreCounts = theLinkScoreMetrics.getScoreCounts(); 149 return metrics; 150 } 151}