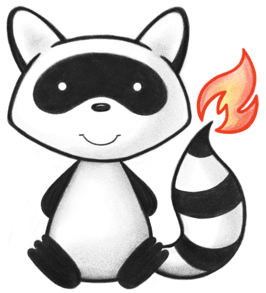
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.model; 021 022import ca.uhn.fhir.rest.api.server.storage.IResourcePersistentId; 023import jakarta.annotation.Nullable; 024 025import java.util.Objects; 026import java.util.StringJoiner; 027 028public class MdmPidTuple<T extends IResourcePersistentId> { 029 private final T myGoldenPid; 030 031 @Nullable 032 private final Integer myGoldenPartitionId; 033 034 private final T mySourcePid; 035 036 @Nullable 037 private final Integer mySourcePartitionId; 038 039 private MdmPidTuple( 040 T theGoldenPid, 041 @Nullable Integer theGoldenPartitionId, 042 T theSourcePid, 043 @Nullable Integer theSourcePartitionId) { 044 myGoldenPid = theGoldenPid; 045 mySourcePid = theSourcePid; 046 myGoldenPartitionId = theGoldenPartitionId; 047 mySourcePartitionId = theSourcePartitionId; 048 } 049 050 public static <P extends IResourcePersistentId> MdmPidTuple<P> fromGoldenAndSource(P theGoldenPid, P theSourcePid) { 051 return new MdmPidTuple<>(theGoldenPid, null, theSourcePid, null); 052 } 053 054 public static <P extends IResourcePersistentId> MdmPidTuple<P> fromGoldenAndSourceAndPartitionIds( 055 P theGoldenPid, Integer theGoldenPartitionId, P theSourcePid, Integer theSourcePartitionId) { 056 return new MdmPidTuple<>(theGoldenPid, theGoldenPartitionId, theSourcePid, theSourcePartitionId); 057 } 058 059 public T getGoldenPid() { 060 return myGoldenPid; 061 } 062 063 @Nullable 064 public Integer getGoldenPartitionId() { 065 return myGoldenPartitionId; 066 } 067 068 public T getSourcePid() { 069 return mySourcePid; 070 } 071 072 @Nullable 073 public Integer getSourcePartitionId() { 074 return mySourcePartitionId; 075 } 076 077 @Override 078 public boolean equals(Object o) { 079 if (this == o) return true; 080 if (o == null || getClass() != o.getClass()) return false; 081 MdmPidTuple<?> that = (MdmPidTuple<?>) o; 082 return Objects.equals(myGoldenPid, that.myGoldenPid) 083 && Objects.equals(myGoldenPartitionId, that.myGoldenPartitionId) 084 && Objects.equals(mySourcePid, that.mySourcePid) 085 && Objects.equals(mySourcePartitionId, that.mySourcePartitionId); 086 } 087 088 @Override 089 public int hashCode() { 090 return Objects.hash(myGoldenPid, myGoldenPartitionId, mySourcePid, mySourcePartitionId); 091 } 092 093 @Override 094 public String toString() { 095 return new StringJoiner(", ", MdmPidTuple.class.getSimpleName() + "[", "]") 096 .add("myGoldenPid=" + myGoldenPid) 097 .add("myGoldenPartitionId=" + myGoldenPartitionId) 098 .add("mySourcePid=" + mySourcePid) 099 .add("mySourcePartitionId=" + mySourcePartitionId) 100 .toString(); 101 } 102}