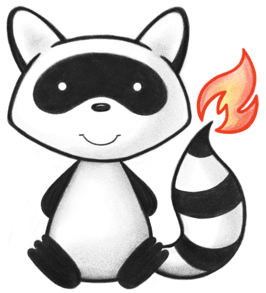
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.model; 021 022import ca.uhn.fhir.mdm.api.IMdmLink; 023import ca.uhn.fhir.rest.server.TransactionLogMessages; 024 025import java.util.ArrayList; 026import java.util.List; 027 028public class MdmTransactionContext { 029 030 public enum OperationType { 031 CREATE_RESOURCE, 032 UPDATE_RESOURCE, 033 SUBMIT_RESOURCE_TO_MDM, 034 QUERY_LINKS, 035 UPDATE_LINK, 036 CREATE_LINK, 037 DUPLICATE_GOLDEN_RESOURCES, 038 NOT_DUPLICATE, 039 MERGE_GOLDEN_RESOURCES, 040 MANUAL_MERGE_GOLDEN_RESOURCES 041 } 042 043 /** 044 * Any MDM methods may add transaction log messages. 045 */ 046 private TransactionLogMessages myTransactionLogMessages; 047 048 private OperationType myRestOperation; 049 050 private String myResourceType; 051 052 /** 053 * Whether or not the currently processed resource is a 'blocked resource'. 054 * This will only be set on matching. 055 */ 056 private boolean myIsBlockedResource; 057 058 private List<IMdmLink> myMdmLinkEvents = new ArrayList<>(); 059 060 public TransactionLogMessages getTransactionLogMessages() { 061 return myTransactionLogMessages; 062 } 063 064 public MdmTransactionContext() {} 065 066 public MdmTransactionContext(OperationType theRestOperation) { 067 myRestOperation = theRestOperation; 068 } 069 070 public MdmTransactionContext(TransactionLogMessages theTransactionLogMessages, OperationType theRestOperation) { 071 this(theRestOperation); 072 myTransactionLogMessages = theTransactionLogMessages; 073 } 074 075 public MdmTransactionContext( 076 TransactionLogMessages theTransactionLogMessages, OperationType theRestOperation, String theResourceType) { 077 this(theTransactionLogMessages, theRestOperation); 078 setResourceType(theResourceType); 079 } 080 081 public void addTransactionLogMessage(String theMessage) { 082 if (myTransactionLogMessages == null) { 083 return; 084 } 085 this.myTransactionLogMessages.addMessage(myTransactionLogMessages, theMessage); 086 } 087 088 public OperationType getRestOperation() { 089 return myRestOperation; 090 } 091 092 public void setTransactionLogMessages(TransactionLogMessages theTransactionLogMessages) { 093 myTransactionLogMessages = theTransactionLogMessages; 094 } 095 096 public void setRestOperation(OperationType theRestOperation) { 097 myRestOperation = theRestOperation; 098 } 099 100 public String getResourceType() { 101 return myResourceType; 102 } 103 104 public void setResourceType(String myResourceType) { 105 this.myResourceType = myResourceType; 106 } 107 108 public List<IMdmLink> getMdmLinks() { 109 return myMdmLinkEvents; 110 } 111 112 public MdmTransactionContext addMdmLink(IMdmLink theMdmLinkEvent) { 113 getMdmLinks().add(theMdmLinkEvent); 114 return this; 115 } 116 117 public void setMdmLinks(List<IMdmLink> theMdmLinkEvents) { 118 myMdmLinkEvents = theMdmLinkEvents; 119 } 120 121 public void setIsBlocked(boolean theIsBlocked) { 122 myIsBlockedResource = theIsBlocked; 123 } 124 125 public boolean getIsBlocked() { 126 return myIsBlockedResource; 127 } 128}