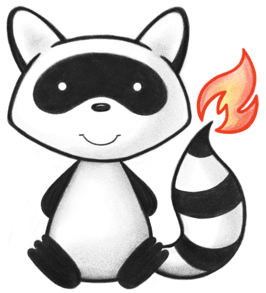
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.model.mdmevents; 021 022import ca.uhn.fhir.model.api.IModelJson; 023import com.fasterxml.jackson.annotation.JsonProperty; 024 025import java.util.ArrayList; 026import java.util.List; 027 028public class MdmHistoryEvent implements IModelJson { 029 030 /** 031 * List of golden resource ids queried. 032 * Can be empty. 033 */ 034 @JsonProperty("goldenResourceIds") 035 private List<String> myGoldenResourceIds; 036 037 /** 038 * List of source ids queried. 039 * Can be empty. 040 */ 041 @JsonProperty("sourceIds") 042 private List<String> mySourceIds; 043 044 /** 045 * The associated link revisions returned from the search. 046 */ 047 @JsonProperty("mdmLinkRevisions") 048 private List<MdmLinkWithRevisionJson> myMdmLinkRevisions; 049 050 public List<String> getGoldenResourceIds() { 051 if (myGoldenResourceIds == null) { 052 myGoldenResourceIds = new ArrayList<>(); 053 } 054 return myGoldenResourceIds; 055 } 056 057 public void setGoldenResourceIds(List<String> theGoldenResourceIds) { 058 myGoldenResourceIds = theGoldenResourceIds; 059 } 060 061 public List<String> getSourceIds() { 062 if (mySourceIds == null) { 063 mySourceIds = new ArrayList<>(); 064 } 065 return mySourceIds; 066 } 067 068 public void setSourceIds(List<String> theSourceIds) { 069 mySourceIds = theSourceIds; 070 } 071 072 public List<MdmLinkWithRevisionJson> getMdmLinkRevisions() { 073 if (myMdmLinkRevisions == null) { 074 myMdmLinkRevisions = new ArrayList<>(); 075 } 076 return myMdmLinkRevisions; 077 } 078 079 public void setMdmLinkRevisions(List<MdmLinkWithRevisionJson> theMdmLinkRevisions) { 080 myMdmLinkRevisions = theMdmLinkRevisions; 081 } 082}