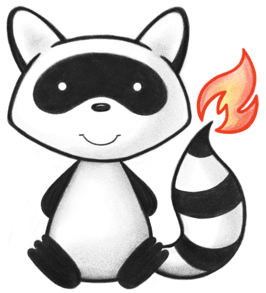
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.model.mdmevents; 021 022import ca.uhn.fhir.mdm.api.MdmLinkSourceEnum; 023import ca.uhn.fhir.mdm.api.MdmMatchResultEnum; 024import ca.uhn.fhir.mdm.rules.json.MdmRulesJson; 025import ca.uhn.fhir.model.api.IModelJson; 026import ca.uhn.fhir.rest.api.server.storage.IResourcePersistentId; 027import com.fasterxml.jackson.annotation.JsonIgnore; 028import com.fasterxml.jackson.annotation.JsonProperty; 029 030import java.util.Date; 031import java.util.HashSet; 032import java.util.Map; 033import java.util.Objects; 034import java.util.Set; 035 036public class MdmLinkJson implements IModelJson { 037 038 /** 039 * Golden resource FhirId 040 */ 041 @JsonProperty("goldenResourceId") 042 private String myGoldenResourceId; 043 044 /** 045 * Source resource FhirId 046 */ 047 @JsonProperty("sourceId") 048 private String mySourceId; 049 050 /** 051 * Golden resource PID 052 */ 053 @JsonIgnore 054 private IResourcePersistentId<?> myGoldenPid; 055 056 /** 057 * Source PID 058 */ 059 @JsonIgnore 060 private IResourcePersistentId<?> mySourcePid; 061 062 /** 063 * Kind of link (MATCH, etc) 064 */ 065 @JsonProperty("matchResult") 066 private MdmMatchResultEnum myMatchResult; 067 068 /** 069 * How the link was constructed (AUTO - by the system, MANUAL - by a user) 070 */ 071 @JsonProperty("linkSource") 072 private MdmLinkSourceEnum myLinkSource; 073 074 @JsonProperty("created") 075 private Date myCreated; 076 077 @JsonProperty("updated") 078 private Date myUpdated; 079 080 @JsonProperty("version") 081 private String myVersion; 082 083 /** 084 * This link was created as a result of an eid match 085 **/ 086 @JsonProperty("eidMatch") 087 private Boolean myEidMatch; 088 089 /** 090 * This link created a new golden resource 091 **/ 092 @JsonProperty("linkCreatedNewGoldenResource") 093 private Boolean myLinkCreatedNewResource; 094 095 @JsonProperty("vector") 096 private Long myVector; 097 098 @JsonProperty("score") 099 private Double myScore; 100 101 @JsonProperty("ruleCount") 102 private Long myRuleCount; 103 104 /** 105 * Matched rules are the rules, as defined in the matchResultMap, that evaluated true 106 * for this link. This property stores the name of the rule, represented by a String, 107 * and the corresponding MatchResultEnum for that rule. 108 */ 109 @JsonProperty(value = "matchedRules") 110 private Set<Map.Entry<String, MdmMatchResultEnum>> myRule = new HashSet<>(); 111 112 public String getGoldenResourceId() { 113 return myGoldenResourceId; 114 } 115 116 public MdmLinkJson setGoldenResourceId(String theGoldenResourceId) { 117 myGoldenResourceId = theGoldenResourceId; 118 return this; 119 } 120 121 public String getSourceId() { 122 return mySourceId; 123 } 124 125 public MdmLinkJson setSourceId(String theSourceId) { 126 mySourceId = theSourceId; 127 return this; 128 } 129 130 public MdmMatchResultEnum getMatchResult() { 131 return myMatchResult; 132 } 133 134 public MdmLinkJson setMatchResult(MdmMatchResultEnum theMatchResult) { 135 myMatchResult = theMatchResult; 136 return this; 137 } 138 139 public MdmLinkSourceEnum getLinkSource() { 140 return myLinkSource; 141 } 142 143 public MdmLinkJson setLinkSource(MdmLinkSourceEnum theLinkSource) { 144 myLinkSource = theLinkSource; 145 return this; 146 } 147 148 public Date getCreated() { 149 return myCreated; 150 } 151 152 public MdmLinkJson setCreated(Date theCreated) { 153 myCreated = theCreated; 154 return this; 155 } 156 157 public Date getUpdated() { 158 return myUpdated; 159 } 160 161 public MdmLinkJson setUpdated(Date theUpdated) { 162 myUpdated = theUpdated; 163 return this; 164 } 165 166 public String getVersion() { 167 return myVersion; 168 } 169 170 public MdmLinkJson setVersion(String theVersion) { 171 myVersion = theVersion; 172 return this; 173 } 174 175 public Boolean getEidMatch() { 176 return myEidMatch; 177 } 178 179 public MdmLinkJson setEidMatch(Boolean theEidMatch) { 180 myEidMatch = theEidMatch; 181 return this; 182 } 183 184 public Boolean getLinkCreatedNewResource() { 185 return myLinkCreatedNewResource; 186 } 187 188 public MdmLinkJson setLinkCreatedNewResource(Boolean theLinkCreatedNewResource) { 189 myLinkCreatedNewResource = theLinkCreatedNewResource; 190 return this; 191 } 192 193 public Long getVector() { 194 return myVector; 195 } 196 197 public MdmLinkJson setVector(Long theVector) { 198 myVector = theVector; 199 return this; 200 } 201 202 public Double getScore() { 203 return myScore; 204 } 205 206 public MdmLinkJson setScore(Double theScore) { 207 myScore = theScore; 208 return this; 209 } 210 211 public Long getRuleCount() { 212 return myRuleCount; 213 } 214 215 public void setRuleCount(Long theRuleCount) { 216 myRuleCount = theRuleCount; 217 } 218 219 public Set<Map.Entry<String, MdmMatchResultEnum>> getRule() { 220 return myRule; 221 } 222 223 public void translateAndSetRule(MdmRulesJson theRule, Long theVector) { 224 myRule = theRule.getMatchedRulesFromVectorMap(theVector); 225 } 226 227 public IResourcePersistentId<?> getGoldenPid() { 228 return myGoldenPid; 229 } 230 231 public void setGoldenPid(IResourcePersistentId<?> theGoldenPid) { 232 myGoldenPid = theGoldenPid; 233 } 234 235 public IResourcePersistentId<?> getSourcePid() { 236 return mySourcePid; 237 } 238 239 public void setSourcePid(IResourcePersistentId<?> theSourcePid) { 240 mySourcePid = theSourcePid; 241 } 242 243 @Override 244 public boolean equals(Object theO) { 245 if (this == theO) return true; 246 if (theO == null || getClass() != theO.getClass()) return false; 247 final MdmLinkJson that = (MdmLinkJson) theO; 248 return Objects.equals(myGoldenResourceId, that.myGoldenResourceId) 249 && Objects.equals(mySourceId, that.mySourceId) 250 && mySourcePid.equals(that.mySourcePid) 251 && myGoldenPid.equals(that.myGoldenPid) 252 && myMatchResult == that.myMatchResult 253 && myLinkSource == that.myLinkSource 254 && Objects.equals(myCreated, that.myCreated) 255 && Objects.equals(myUpdated, that.myUpdated) 256 && Objects.equals(myVersion, that.myVersion) 257 && Objects.equals(myEidMatch, that.myEidMatch) 258 && Objects.equals(myLinkCreatedNewResource, that.myLinkCreatedNewResource) 259 && Objects.equals(myVector, that.myVector) 260 && Objects.equals(myScore, that.myScore) 261 && Objects.equals(myRuleCount, that.myRuleCount); 262 } 263 264 @Override 265 public int hashCode() { 266 return Objects.hash( 267 myGoldenResourceId, 268 mySourceId, 269 mySourcePid, 270 myGoldenPid, 271 myMatchResult, 272 myLinkSource, 273 myCreated, 274 myUpdated, 275 myVersion, 276 myEidMatch, 277 myLinkCreatedNewResource, 278 myVector, 279 myScore, 280 myRuleCount); 281 } 282 283 @Override 284 public String toString() { 285 return "MdmLinkJson{" 286 + "myGoldenResourceId='" + myGoldenResourceId + '\'' 287 + ", myGoldenPid='" + myGoldenPid + '\'' 288 + ", mySourceId='" + mySourceId + '\'' 289 + ", mySourcePid='" + mySourcePid + '\'' 290 + ", myMatchResult=" + myMatchResult 291 + ", myLinkSource=" + myLinkSource 292 + ", myCreated=" + myCreated 293 + ", myUpdated=" + myUpdated 294 + ", myVersion='" + myVersion + '\'' 295 + ", myEidMatch=" + myEidMatch 296 + ", myLinkCreatedNewResource=" + myLinkCreatedNewResource 297 + ", myVector=" + myVector 298 + ", myScore=" + myScore 299 + ", myRuleCount=" + myRuleCount 300 + '}'; 301 } 302}