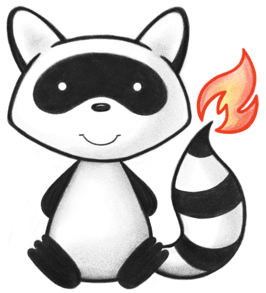
001package ca.uhn.fhir.mdm.model.mdmevents; 002 003/*- 004 * #%L 005 * HAPI FHIR - Master Data Management 006 * %% 007 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 008 * %% 009 * Licensed under the Apache License, Version 2.0 (the "License"); 010 * you may not use this file except in compliance with the License. 011 * You may obtain a copy of the License at 012 * 013 * http://www.apache.org/licenses/LICENSE-2.0 014 * 015 * Unless required by applicable law or agreed to in writing, software 016 * distributed under the License is distributed on an "AS IS" BASIS, 017 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 018 * See the License for the specific language governing permissions and 019 * limitations under the License. 020 * #L% 021 */ 022 023import ca.uhn.fhir.model.api.IModelJson; 024import com.fasterxml.jackson.annotation.JsonProperty; 025import org.apache.commons.lang3.builder.ToStringBuilder; 026 027import java.util.Date; 028import java.util.Objects; 029 030public class MdmLinkWithRevisionJson implements IModelJson { 031 @JsonProperty(value = "mdmLink", required = true) 032 MdmLinkJson myMdmLink; 033 034 @JsonProperty(value = "revisionNumber", required = true) 035 Long myRevisionNumber; 036 037 @JsonProperty(value = "revisionTimestamp", required = true) 038 Date myRevisionTimestamp; 039 040 public MdmLinkWithRevisionJson(MdmLinkJson theMdmLink, Long theRevisionNumber, Date theRevisionTimestamp) { 041 myMdmLink = theMdmLink; 042 myRevisionNumber = theRevisionNumber; 043 myRevisionTimestamp = theRevisionTimestamp; 044 } 045 046 public MdmLinkJson getMdmLink() { 047 return myMdmLink; 048 } 049 050 public Long getRevisionNumber() { 051 return myRevisionNumber; 052 } 053 054 public Date getRevisionTimestamp() { 055 return myRevisionTimestamp; 056 } 057 058 @Override 059 public boolean equals(Object theO) { 060 if (this == theO) return true; 061 if (theO == null || getClass() != theO.getClass()) return false; 062 final MdmLinkWithRevisionJson that = (MdmLinkWithRevisionJson) theO; 063 return myMdmLink.equals(that.myMdmLink) 064 && myRevisionNumber.equals(that.myRevisionNumber) 065 && myRevisionTimestamp.equals(that.myRevisionTimestamp); 066 } 067 068 @Override 069 public int hashCode() { 070 return Objects.hash(myMdmLink, myRevisionNumber, myRevisionTimestamp); 071 } 072 073 @Override 074 public String toString() { 075 return new ToStringBuilder(this) 076 .append("myMdmLink", myMdmLink) 077 .append("myRevisionNumber", myRevisionNumber) 078 .append("myRevisionTimestamp", myRevisionTimestamp) 079 .toString(); 080 } 081}