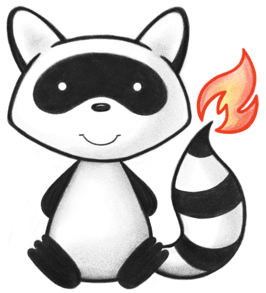
001package ca.uhn.fhir.mdm.provider; 002 003/*- 004 * #%L 005 * HAPI FHIR - Master Data Management 006 * %% 007 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 008 * %% 009 * Licensed under the Apache License, Version 2.0 (the "License"); 010 * you may not use this file except in compliance with the License. 011 * You may obtain a copy of the License at 012 * 013 * http://www.apache.org/licenses/LICENSE-2.0 014 * 015 * Unless required by applicable law or agreed to in writing, software 016 * distributed under the License is distributed on an "AS IS" BASIS, 017 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 018 * See the License for the specific language governing permissions and 019 * limitations under the License. 020 * #L% 021 */ 022 023import ca.uhn.fhir.context.FhirContext; 024import ca.uhn.fhir.interceptor.api.HookParams; 025import ca.uhn.fhir.interceptor.api.IInterceptorBroadcaster; 026import ca.uhn.fhir.interceptor.api.Pointcut; 027import ca.uhn.fhir.mdm.api.IMdmControllerSvc; 028import ca.uhn.fhir.mdm.api.params.MdmHistorySearchParameters; 029import ca.uhn.fhir.mdm.model.mdmevents.MdmHistoryEvent; 030import ca.uhn.fhir.mdm.model.mdmevents.MdmLinkWithRevisionJson; 031import ca.uhn.fhir.model.api.annotation.Description; 032import ca.uhn.fhir.rest.annotation.Operation; 033import ca.uhn.fhir.rest.annotation.OperationParam; 034import ca.uhn.fhir.rest.api.server.RequestDetails; 035import ca.uhn.fhir.rest.server.provider.ProviderConstants; 036import ca.uhn.fhir.rest.server.servlet.ServletRequestDetails; 037import ca.uhn.fhir.util.ParametersUtil; 038import org.hl7.fhir.instance.model.api.IBaseParameters; 039import org.hl7.fhir.instance.model.api.IPrimitiveType; 040import org.slf4j.Logger; 041 042import java.util.List; 043import java.util.stream.Collectors; 044 045import static org.apache.commons.collections4.CollectionUtils.isNotEmpty; 046import static org.slf4j.LoggerFactory.getLogger; 047 048public class MdmLinkHistoryProviderDstu3Plus extends BaseMdmProvider { 049 private static final Logger ourLog = getLogger(MdmLinkHistoryProviderDstu3Plus.class); 050 051 private final IInterceptorBroadcaster myInterceptorBroadcaster; 052 053 public MdmLinkHistoryProviderDstu3Plus( 054 FhirContext theFhirContext, 055 IMdmControllerSvc theMdmControllerSvc, 056 IInterceptorBroadcaster theIInterceptorBroadcaster) { 057 super(theFhirContext, theMdmControllerSvc); 058 myInterceptorBroadcaster = theIInterceptorBroadcaster; 059 } 060 061 @Operation(name = ProviderConstants.MDM_LINK_HISTORY, idempotent = true) 062 public IBaseParameters historyLinks( 063 @Description(value = "The id of the Golden Resource (e.g. Golden Patient Resource).") 064 @OperationParam( 065 name = ProviderConstants.MDM_QUERY_LINKS_GOLDEN_RESOURCE_ID, 066 min = 0, 067 max = OperationParam.MAX_UNLIMITED, 068 typeName = "string") 069 List<IPrimitiveType<String>> theMdmGoldenResourceIds, 070 @Description(value = "The id of the source resource (e.g. Patient resource).") 071 @OperationParam( 072 name = ProviderConstants.MDM_QUERY_LINKS_RESOURCE_ID, 073 min = 0, 074 max = OperationParam.MAX_UNLIMITED, 075 typeName = "string") 076 List<IPrimitiveType<String>> theResourceIds, 077 ServletRequestDetails theRequestDetails) { 078 validateMdmLinkHistoryParameters(theMdmGoldenResourceIds, theResourceIds); 079 080 final List<String> goldenResourceIdsToUse = 081 convertToStringsIncludingCommaDelimitedIfNotNull(theMdmGoldenResourceIds); 082 final List<String> resourceIdsToUse = convertToStringsIncludingCommaDelimitedIfNotNull(theResourceIds); 083 084 final IBaseParameters retVal = ParametersUtil.newInstance(myFhirContext); 085 086 final MdmHistorySearchParameters mdmHistorySearchParameters = new MdmHistorySearchParameters() 087 .setGoldenResourceIds(goldenResourceIdsToUse) 088 .setSourceIds(resourceIdsToUse); 089 090 final List<MdmLinkWithRevisionJson> mdmLinkRevisionsFromSvc = 091 myMdmControllerSvc.queryLinkHistory(mdmHistorySearchParameters, theRequestDetails); 092 093 parametersFromMdmLinkRevisions(retVal, mdmLinkRevisionsFromSvc, theRequestDetails); 094 095 if (myInterceptorBroadcaster.hasHooks(Pointcut.MDM_POST_LINK_HISTORY)) { 096 // MDM_POST_LINK_HISTORY hook 097 MdmHistoryEvent historyEvent = new MdmHistoryEvent(); 098 historyEvent.setMdmLinkRevisions(mdmLinkRevisionsFromSvc); 099 if (isNotEmpty(theResourceIds)) { 100 historyEvent.setSourceIds(theResourceIds.stream() 101 .map(IPrimitiveType::getValueAsString) 102 .collect(Collectors.toList())); 103 } 104 if (isNotEmpty(theMdmGoldenResourceIds)) { 105 historyEvent.setGoldenResourceIds(theMdmGoldenResourceIds.stream() 106 .map(IPrimitiveType::getValueAsString) 107 .collect(Collectors.toList())); 108 } 109 110 HookParams params = new HookParams(); 111 params.add(RequestDetails.class, theRequestDetails); 112 params.add(MdmHistoryEvent.class, historyEvent); 113 myInterceptorBroadcaster.callHooks(Pointcut.MDM_POST_LINK_HISTORY, params); 114 } 115 116 return retVal; 117 } 118}