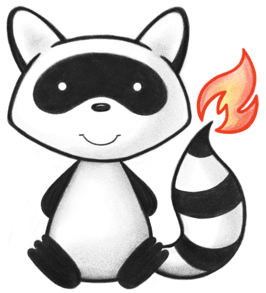
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.provider; 021 022import ca.uhn.fhir.context.ConfigurationException; 023import ca.uhn.fhir.context.FhirContext; 024import ca.uhn.fhir.i18n.Msg; 025import ca.uhn.fhir.interceptor.api.IInterceptorBroadcaster; 026import ca.uhn.fhir.jpa.api.config.JpaStorageSettings; 027import ca.uhn.fhir.mdm.api.IMdmControllerSvc; 028import ca.uhn.fhir.mdm.api.IMdmSettings; 029import ca.uhn.fhir.mdm.api.IMdmSubmitSvc; 030import ca.uhn.fhir.rest.server.provider.ResourceProviderFactory; 031import jakarta.annotation.PreDestroy; 032import org.springframework.beans.factory.annotation.Autowired; 033import org.springframework.stereotype.Service; 034 035import java.util.function.Supplier; 036 037@Service 038public class MdmProviderLoader { 039 @Autowired 040 protected FhirContext myFhirContext; 041 042 @Autowired 043 protected ResourceProviderFactory myResourceProviderFactory; 044 045 @Autowired 046 private MdmControllerHelper myMdmControllerHelper; 047 048 @Autowired 049 private IMdmControllerSvc myMdmControllerSvc; 050 051 @Autowired 052 private IMdmSubmitSvc myMdmSubmitSvc; 053 054 @Autowired 055 private IMdmSettings myMdmSettings; 056 057 @Autowired 058 private JpaStorageSettings myStorageSettings; 059 060 @Autowired 061 private IInterceptorBroadcaster myInterceptorBroadcaster; 062 063 protected Supplier<Object> myMdmProviderSupplier; 064 private Supplier<Object> myPatientMatchProviderSupplier; 065 private Supplier<Object> myMdmHistoryProviderSupplier; 066 067 public void loadPatientMatchProvider() { 068 switch (myFhirContext.getVersion().getVersion()) { 069 case DSTU3: 070 case R4: 071 case R5: 072 // We store the supplier so that removeSupplier works properly 073 myPatientMatchProviderSupplier = () -> new PatientMatchProvider(myMdmControllerHelper); 074 myResourceProviderFactory.addSupplier(myPatientMatchProviderSupplier); 075 break; 076 default: 077 throw new ConfigurationException(Msg.code(2574) + "Patient/$match not supported for FHIR version " 078 + myFhirContext.getVersion().getVersion()); 079 } 080 } 081 082 public void loadProvider() { 083 switch (myFhirContext.getVersion().getVersion()) { 084 case DSTU3: 085 case R4: 086 case R5: 087 // We store the supplier so that removeSupplier works properly 088 myMdmProviderSupplier = () -> new MdmProviderDstu3Plus( 089 myFhirContext, 090 myMdmControllerSvc, 091 myMdmControllerHelper, 092 myMdmSubmitSvc, 093 myInterceptorBroadcaster, 094 myMdmSettings); 095 myResourceProviderFactory.addSupplier(myMdmProviderSupplier); 096 if (myStorageSettings.isNonResourceDbHistoryEnabled()) { 097 myMdmHistoryProviderSupplier = () -> new MdmLinkHistoryProviderDstu3Plus( 098 myFhirContext, myMdmControllerSvc, myInterceptorBroadcaster); 099 myResourceProviderFactory.addSupplier(myMdmHistoryProviderSupplier); 100 } 101 break; 102 default: 103 throw new ConfigurationException(Msg.code(1497) + "MDM not supported for FHIR version " 104 + myFhirContext.getVersion().getVersion()); 105 } 106 } 107 108 @PreDestroy 109 public void unloadProvider() { 110 if (myMdmProviderSupplier != null) { 111 myResourceProviderFactory.removeSupplier(myMdmProviderSupplier); 112 } 113 if (myMdmHistoryProviderSupplier != null) { 114 myResourceProviderFactory.removeSupplier(myMdmHistoryProviderSupplier); 115 } 116 if (myPatientMatchProviderSupplier != null) { 117 myResourceProviderFactory.removeSupplier(myPatientMatchProviderSupplier); 118 } 119 } 120}