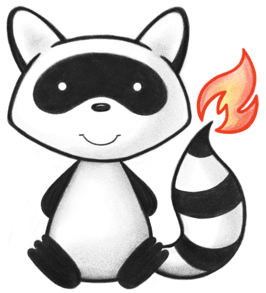
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.rules.config; 021 022import ca.uhn.fhir.mdm.api.IMdmRuleValidator; 023import ca.uhn.fhir.mdm.api.IMdmSettings; 024import ca.uhn.fhir.mdm.api.MdmModeEnum; 025import ca.uhn.fhir.mdm.rules.json.MdmRulesJson; 026import ca.uhn.fhir.util.JsonUtil; 027import org.springframework.beans.factory.annotation.Autowired; 028import org.springframework.stereotype.Component; 029 030import java.io.IOException; 031 032import static ca.uhn.fhir.mdm.api.MdmModeEnum.MATCH_AND_LINK; 033 034@Component 035public class MdmSettings implements IMdmSettings { 036 public static final int DEFAULT_CANDIDATE_SEARCH_LIMIT = 10000; 037 private final IMdmRuleValidator myMdmRuleValidator; 038 039 private boolean myEnabled; 040 private final int myConcurrentConsumers = MDM_DEFAULT_CONCURRENT_CONSUMERS; 041 private String myScriptText; 042 private String mySurvivorshipRules; 043 private MdmRulesJson myMdmRules; 044 private boolean myPreventEidUpdates; 045 private String myGoldenResourcePartitionName; 046 private boolean mySearchAllPartitionForMatch = false; 047 private boolean myShouldAutoDeleteGoldenResources = true; 048 private MdmModeEnum myMdmMode = MATCH_AND_LINK; 049 050 /** 051 * If disabled, the underlying MDM system will operate under the following assumptions: 052 * <p> 053 * 1. Source resource may have more than 1 EID of the same system simultaneously. 054 * 2. During linking, incoming patient EIDs will be merged with existing Golden Resource EIDs. 055 */ 056 private boolean myPreventMultipleEids; 057 058 /** 059 * When searching for matching candidates, this is the maximum number of candidates that will be retrieved. If the 060 * number matched is equal to or higher than this, then an exception will be thrown and candidate matching will be aborted 061 */ 062 private int myCandidateSearchLimit = DEFAULT_CANDIDATE_SEARCH_LIMIT; 063 064 @Autowired 065 public MdmSettings(IMdmRuleValidator theMdmRuleValidator) { 066 myMdmRuleValidator = theMdmRuleValidator; 067 } 068 069 @Override 070 public boolean isEnabled() { 071 return myEnabled; 072 } 073 074 public MdmSettings setEnabled(boolean theEnabled) { 075 myEnabled = theEnabled; 076 return this; 077 } 078 079 @Override 080 public int getConcurrentConsumers() { 081 return myConcurrentConsumers; 082 } 083 084 public String getScriptText() { 085 return myScriptText; 086 } 087 088 public MdmSettings setScriptText(String theScriptText) throws IOException { 089 myScriptText = theScriptText; 090 setMdmRules(JsonUtil.deserialize(theScriptText, MdmRulesJson.class)); 091 return this; 092 } 093 094 @Override 095 public MdmRulesJson getMdmRules() { 096 return myMdmRules; 097 } 098 099 @Override 100 public boolean isPreventEidUpdates() { 101 return myPreventEidUpdates; 102 } 103 104 public MdmSettings setPreventEidUpdates(boolean thePreventEidUpdates) { 105 myPreventEidUpdates = thePreventEidUpdates; 106 return this; 107 } 108 109 public MdmSettings setMdmRules(MdmRulesJson theMdmRules) { 110 myMdmRuleValidator.validate(theMdmRules); 111 myMdmRules = theMdmRules; 112 return this; 113 } 114 115 public boolean isPreventMultipleEids() { 116 return myPreventMultipleEids; 117 } 118 119 @Override 120 public String getRuleVersion() { 121 return myMdmRules.getVersion(); 122 } 123 124 public MdmSettings setPreventMultipleEids(boolean thePreventMultipleEids) { 125 myPreventMultipleEids = thePreventMultipleEids; 126 return this; 127 } 128 129 @Override 130 public String getSurvivorshipRules() { 131 return mySurvivorshipRules; 132 } 133 134 public void setSurvivorshipRules(String theSurvivorshipRules) { 135 mySurvivorshipRules = theSurvivorshipRules; 136 } 137 138 @Override 139 public int getCandidateSearchLimit() { 140 return myCandidateSearchLimit; 141 } 142 143 public void setCandidateSearchLimit(int theCandidateSearchLimit) { 144 myCandidateSearchLimit = theCandidateSearchLimit; 145 } 146 147 @Override 148 public String getGoldenResourcePartitionName() { 149 return myGoldenResourcePartitionName; 150 } 151 152 @Override 153 public void setGoldenResourcePartitionName(String theGoldenResourcePartitionName) { 154 myGoldenResourcePartitionName = theGoldenResourcePartitionName; 155 } 156 157 @Override 158 public boolean getSearchAllPartitionForMatch() { 159 return mySearchAllPartitionForMatch; 160 } 161 162 @Override 163 public void setSearchAllPartitionForMatch(boolean theSearchAllPartitionForMatch) { 164 mySearchAllPartitionForMatch = theSearchAllPartitionForMatch; 165 } 166 167 @Override 168 public boolean isAutoExpungeGoldenResources() { 169 return myShouldAutoDeleteGoldenResources; 170 } 171 172 @Override 173 public void setAutoExpungeGoldenResources(boolean theShouldAutoExpunge) { 174 myShouldAutoDeleteGoldenResources = theShouldAutoExpunge; 175 } 176 177 public void setMdmMode(MdmModeEnum theMdmMode) { 178 myMdmMode = theMdmMode; 179 } 180 181 @Override 182 public MdmModeEnum getMode() { 183 return myMdmMode; 184 } 185}