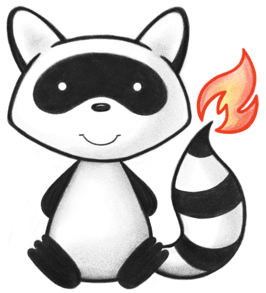
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.rules.json; 021 022import ca.uhn.fhir.mdm.rules.matcher.models.MatchTypeEnum; 023import ca.uhn.fhir.model.api.IModelJson; 024import com.fasterxml.jackson.annotation.JsonProperty; 025import jakarta.annotation.Nonnull; 026 027/** 028 * Contains all business data for determining if a match exists on a particular field, given: 029 * <p></p> 030 * 1. A {@link MatchTypeEnum} which determines the actual similarity values. 031 * 2. A given resource type (e.g. Patient) 032 * 3. A given FHIRPath expression for finding the particular primitive to be used for comparison. (e.g. name.given) 033 */ 034public class MdmFieldMatchJson implements IModelJson { 035 @JsonProperty(value = "name", required = true) 036 String myName; 037 038 @JsonProperty(value = "resourceType", required = true) 039 String myResourceType; 040 041 @JsonProperty(value = "resourcePath", required = false) 042 String myResourcePath; 043 044 @JsonProperty(value = "fhirPath", required = false) 045 String myFhirPath; 046 047 @JsonProperty(value = "matcher", required = false) 048 MdmMatcherJson myMatcher; 049 050 @JsonProperty(value = "similarity", required = false) 051 MdmSimilarityJson mySimilarity; 052 053 public String getResourceType() { 054 return myResourceType; 055 } 056 057 public MdmFieldMatchJson setResourceType(String theResourceType) { 058 myResourceType = theResourceType; 059 return this; 060 } 061 062 public String getResourcePath() { 063 return myResourcePath; 064 } 065 066 public MdmFieldMatchJson setResourcePath(String theResourcePath) { 067 myResourcePath = theResourcePath; 068 return this; 069 } 070 071 public String getName() { 072 return myName; 073 } 074 075 public MdmFieldMatchJson setName(@Nonnull String theName) { 076 myName = theName; 077 return this; 078 } 079 080 public MdmMatcherJson getMatcher() { 081 return myMatcher; 082 } 083 084 public MdmFieldMatchJson setMatcher(MdmMatcherJson theMatcher) { 085 myMatcher = theMatcher; 086 return this; 087 } 088 089 public MdmSimilarityJson getSimilarity() { 090 return mySimilarity; 091 } 092 093 public MdmFieldMatchJson setSimilarity(MdmSimilarityJson theSimilarity) { 094 mySimilarity = theSimilarity; 095 return this; 096 } 097 098 public String getFhirPath() { 099 return myFhirPath; 100 } 101 102 public MdmFieldMatchJson setFhirPath(String theFhirPath) { 103 myFhirPath = theFhirPath; 104 return this; 105 } 106}