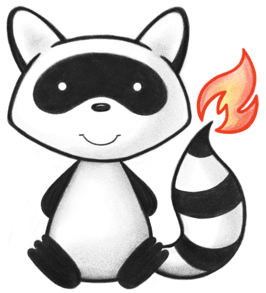
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.rules.json; 021 022import ca.uhn.fhir.model.api.IModelJson; 023import ca.uhn.fhir.rest.param.TokenParamModifier; 024import com.fasterxml.jackson.annotation.JsonProperty; 025 026/** 027 * This class, unlike {@link MdmResourceSearchParamJson}, is responsible for doing inclusions during MDM 028 * candidate searching. e.g. When doing candidate matching, only consider candidates that match all 029 * MdmFilterSearchParams. 030 */ 031public class MdmFilterSearchParamJson implements IModelJson { 032 @JsonProperty(value = "resourceType", required = true) 033 String myResourceType; 034 035 @JsonProperty(value = "searchParam", required = true) 036 String mySearchParam; 037 038 @JsonProperty(value = "qualifier", required = true) 039 TokenParamModifier myTokenParamModifier; 040 041 @JsonProperty(value = "fixedValue", required = true) 042 String myFixedValue; 043 044 public String getResourceType() { 045 return myResourceType; 046 } 047 048 public MdmFilterSearchParamJson setResourceType(String theResourceType) { 049 myResourceType = theResourceType; 050 return this; 051 } 052 053 public String getSearchParam() { 054 return mySearchParam; 055 } 056 057 public MdmFilterSearchParamJson setSearchParam(String theSearchParam) { 058 mySearchParam = theSearchParam; 059 return this; 060 } 061 062 public TokenParamModifier getTokenParamModifier() { 063 return myTokenParamModifier; 064 } 065 066 public MdmFilterSearchParamJson setTokenParamModifier(TokenParamModifier theTokenParamModifier) { 067 myTokenParamModifier = theTokenParamModifier; 068 return this; 069 } 070 071 public String getFixedValue() { 072 return myFixedValue; 073 } 074 075 public MdmFilterSearchParamJson setFixedValue(String theFixedValue) { 076 myFixedValue = theFixedValue; 077 return this; 078 } 079 080 public String getTokenParamModifierAsString() { 081 return myTokenParamModifier == null ? "" : myTokenParamModifier.getValue(); 082 } 083}