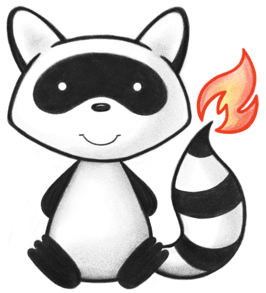
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.rules.json; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.mdm.api.MdmMatchEvaluation; 024import ca.uhn.fhir.mdm.rules.similarity.MdmSimilarityEnum; 025import ca.uhn.fhir.model.api.IModelJson; 026import com.fasterxml.jackson.annotation.JsonProperty; 027import jakarta.annotation.Nullable; 028import org.hl7.fhir.instance.model.api.IBase; 029 030public class MdmSimilarityJson implements IModelJson { 031 @JsonProperty(value = "algorithm", required = true) 032 MdmSimilarityEnum myAlgorithm; 033 034 @JsonProperty(value = "matchThreshold", required = true) 035 Double myMatchThreshold; 036 037 /** 038 * For String value types, should the values be normalized (case, accents) before they are compared 039 */ 040 @JsonProperty(value = "exact") 041 boolean myExact; 042 043 public MdmSimilarityEnum getAlgorithm() { 044 return myAlgorithm; 045 } 046 047 public MdmSimilarityJson setAlgorithm(MdmSimilarityEnum theAlgorithm) { 048 myAlgorithm = theAlgorithm; 049 return this; 050 } 051 052 @Nullable 053 public Double getMatchThreshold() { 054 return myMatchThreshold; 055 } 056 057 public MdmSimilarityJson setMatchThreshold(double theMatchThreshold) { 058 myMatchThreshold = theMatchThreshold; 059 return this; 060 } 061 062 public boolean getExact() { 063 return myExact; 064 } 065 066 public MdmSimilarityJson setExact(boolean theExact) { 067 myExact = theExact; 068 return this; 069 } 070 071 public MdmMatchEvaluation match(FhirContext theFhirContext, IBase theLeftValue, IBase theRightValue) { 072 return myAlgorithm.match(theFhirContext, theLeftValue, theRightValue, myExact, myMatchThreshold); 073 } 074}