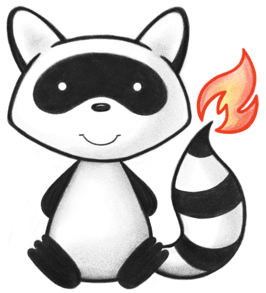
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.rules.matcher.fieldmatchers; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.i18n.Msg; 024import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 025import org.hl7.fhir.instance.model.api.IBase; 026import org.hl7.fhir.r4.model.DateTimeType; 027 028/** 029 * A wrapper class for datetimes of ambiguous fhir version 030 */ 031public class DateTimeWrapper { 032 033 /** 034 * The precision of this datetime object 035 */ 036 private final TemporalPrecisionEnum myPrecision; 037 038 /** 039 * The string value with current precision 040 */ 041 private final String myValueAsString; 042 043 public DateTimeWrapper(FhirContext theFhirContext, IBase theDate) { 044 if (theDate instanceof org.hl7.fhir.dstu3.model.BaseDateTimeType) { 045 org.hl7.fhir.dstu3.model.BaseDateTimeType dstu3Date = (org.hl7.fhir.dstu3.model.BaseDateTimeType) theDate; 046 myPrecision = dstu3Date.getPrecision(); 047 myValueAsString = dstu3Date.getValueAsString(); 048 } else if (theDate instanceof org.hl7.fhir.r4.model.BaseDateTimeType) { 049 org.hl7.fhir.r4.model.BaseDateTimeType r4Date = (org.hl7.fhir.r4.model.BaseDateTimeType) theDate; 050 myPrecision = r4Date.getPrecision(); 051 myValueAsString = r4Date.getValueAsString(); 052 } else if (theDate instanceof org.hl7.fhir.r5.model.BaseDateTimeType) { 053 org.hl7.fhir.r5.model.BaseDateTimeType r5Date = (org.hl7.fhir.r5.model.BaseDateTimeType) theDate; 054 myPrecision = r5Date.getPrecision(); 055 myValueAsString = r5Date.getValueAsString(); 056 } else { 057 // we should consider changing this error so we don't need the fhir context at all 058 throw new UnsupportedOperationException(Msg.code(1520) + "Version not supported: " 059 + theFhirContext.getVersion().getVersion()); 060 } 061 } 062 063 public TemporalPrecisionEnum getPrecision() { 064 return myPrecision; 065 } 066 067 public String getValueAsStringWithPrecision(TemporalPrecisionEnum thePrecision) { 068 // we are using an R4 DateTypes because all datetime strings are the same for all fhir versions 069 // (and so it won't matter here) 070 // we just want the string at a specific precision 071 DateTimeType dateTimeType = new DateTimeType(myValueAsString); 072 dateTimeType.setPrecision(thePrecision); 073 return dateTimeType.getValueAsString(); 074 } 075 076 public String getValueAsString() { 077 return myValueAsString; 078 } 079}