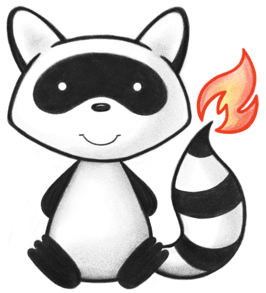
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.rules.matcher.fieldmatchers; 021 022import ca.uhn.fhir.jpa.nickname.INicknameSvc; 023import ca.uhn.fhir.mdm.rules.json.MdmMatcherJson; 024import ca.uhn.fhir.mdm.rules.matcher.models.IMdmFieldMatcher; 025import ca.uhn.fhir.mdm.rules.matcher.util.StringMatcherUtils; 026import org.hl7.fhir.instance.model.api.IBase; 027import org.hl7.fhir.instance.model.api.IPrimitiveType; 028 029import java.util.Collection; 030import java.util.Locale; 031 032public class NicknameMatcher implements IMdmFieldMatcher { 033 private final INicknameSvc myNicknameSvc; 034 035 public NicknameMatcher(INicknameSvc theNicknameSvc) { 036 myNicknameSvc = theNicknameSvc; 037 } 038 039 public boolean matches(String theLeftString, String theRightString) { 040 String leftString = theLeftString.toLowerCase(Locale.ROOT); 041 String rightString = theRightString.toLowerCase(Locale.ROOT); 042 043 Collection<String> leftNames = myNicknameSvc.getEquivalentNames(leftString); 044 if (leftNames.contains(rightString)) { 045 return true; 046 } 047 048 Collection<String> rightNames = myNicknameSvc.getEquivalentNames(rightString); 049 return rightNames.contains(leftString); 050 } 051 052 @Override 053 public boolean matches(IBase theLeftBase, IBase theRightBase, MdmMatcherJson theParams) { 054 if (theLeftBase instanceof IPrimitiveType && theRightBase instanceof IPrimitiveType) { 055 String leftString = StringMatcherUtils.extractString((IPrimitiveType<?>) theLeftBase, theParams.getExact()); 056 String rightString = 057 StringMatcherUtils.extractString((IPrimitiveType<?>) theRightBase, theParams.getExact()); 058 059 return matches(leftString, rightString); 060 } 061 return false; 062 } 063}