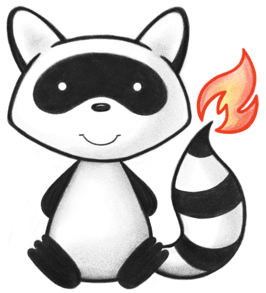
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.svc; 021 022import ca.uhn.fhir.interceptor.model.RequestPartitionId; 023import ca.uhn.fhir.jpa.api.svc.IIdHelperService; 024import ca.uhn.fhir.mdm.api.MdmMatchResultEnum; 025import ca.uhn.fhir.mdm.dao.IMdmLinkDao; 026import ca.uhn.fhir.rest.api.server.storage.IResourcePersistentId; 027import org.hl7.fhir.instance.model.api.IBaseResource; 028import org.slf4j.Logger; 029import org.slf4j.LoggerFactory; 030import org.springframework.beans.factory.annotation.Autowired; 031import org.springframework.stereotype.Service; 032 033@Service 034public class MdmLinkDeleteSvc { 035 private static final Logger ourLog = LoggerFactory.getLogger(MdmLinkDeleteSvc.class); 036 037 @Autowired 038 private IMdmLinkDao myMdmLinkDao; 039 040 @Autowired 041 private IIdHelperService myIdHelperService; 042 043 /** 044 * Delete all {@link ca.uhn.fhir.mdm.api.IMdmLink} records that implements this interface. (Used by Expunge.) 045 * 046 * @param theResource 047 * @return the number of records deleted 048 */ 049 public int deleteWithAnyReferenceTo(IBaseResource theResource) { 050 IResourcePersistentId pid = myIdHelperService.getPidOrThrowException( 051 RequestPartitionId.allPartitions(), theResource.getIdElement()); 052 int removed = myMdmLinkDao.deleteWithAnyReferenceToPid(pid); 053 if (removed > 0) { 054 ourLog.info( 055 "Removed {} MDM links with references to {}", 056 removed, 057 theResource.getIdElement().toVersionless()); 058 } 059 return removed; 060 } 061 062 public int deleteNonRedirectWithAnyReferenceTo(IBaseResource theResource) { 063 IResourcePersistentId pid = myIdHelperService.getPidOrThrowException( 064 RequestPartitionId.allPartitions(), theResource.getIdElement()); 065 int removed = myMdmLinkDao.deleteWithAnyReferenceToPidAndMatchResultNot(pid, MdmMatchResultEnum.REDIRECT); 066 if (removed > 0) { 067 ourLog.info( 068 "Removed {} non-redirect MDM links with references to {}", 069 removed, 070 theResource.getIdElement().toVersionless()); 071 } 072 return removed; 073 } 074}