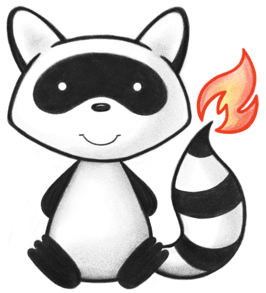
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.svc; 021 022import org.hl7.fhir.instance.model.api.IIdType; 023 024import java.util.HashMap; 025import java.util.HashSet; 026import java.util.Map; 027import java.util.Optional; 028import java.util.Set; 029 030/** 031 * Result object for {@link MdmSearchExpansionSvc} 032 * 033 * @since 8.0.0 034 */ 035public class MdmSearchExpansionResults { 036 037 private final Set<IIdType> myOriginalIdToExpandedId = new HashSet<>(); 038 private final Map<IIdType, IIdType> myExpandedIdToOriginalId = new HashMap<>(); 039 040 void addExpandedId(IIdType theOriginalId, IIdType theExpandedId) { 041 assert isRemapCandidate(theOriginalId) : theOriginalId.getValue(); 042 myOriginalIdToExpandedId.add(theOriginalId); 043 myExpandedIdToOriginalId.put(theExpandedId, theOriginalId); 044 } 045 046 public Optional<IIdType> getOriginalIdForExpandedId(IIdType theId) { 047 assert isRemapCandidate(theId) : theId.getValue(); 048 049 // If we have this ID in the OriginalId map, it was explicitly 050 // searched for, so even if it's also an expanded ID we don't 051 // want to consider it as one 052 if (myOriginalIdToExpandedId.contains(theId)) { 053 return Optional.empty(); 054 } 055 056 IIdType originalId = myExpandedIdToOriginalId.get(theId); 057 return Optional.ofNullable(originalId); 058 } 059 060 public static boolean isRemapCandidate(IIdType theId) { 061 return theId != null 062 && !theId.isLocal() 063 && !theId.isUuid() 064 && theId.hasResourceType() 065 && theId.hasIdPart() 066 && theId.getValue().equals(theId.toUnqualifiedVersionless().getValue()); 067 } 068}