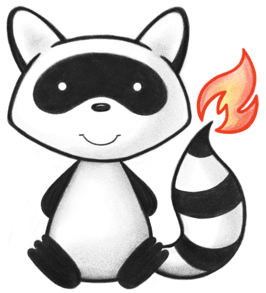
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.svc; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.context.RuntimeResourceDefinition; 024import ca.uhn.fhir.context.RuntimeSearchParam; 025import ca.uhn.fhir.jpa.api.dao.DaoRegistry; 026import ca.uhn.fhir.jpa.api.dao.IFhirResourceDao; 027import ca.uhn.fhir.jpa.dao.ISearchBuilder; 028import ca.uhn.fhir.jpa.dao.SearchBuilderFactory; 029import ca.uhn.fhir.jpa.searchparam.MatchUrlService; 030import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 031import ca.uhn.fhir.jpa.searchparam.extractor.SearchParamExtractorService; 032import ca.uhn.fhir.rest.server.util.ISearchParamRegistry; 033import ca.uhn.fhir.util.SearchParameterUtil; 034import jakarta.annotation.Nullable; 035import org.apache.commons.lang3.StringUtils; 036import org.hl7.fhir.instance.model.api.IBaseResource; 037import org.springframework.beans.factory.annotation.Autowired; 038import org.springframework.stereotype.Service; 039 040import java.util.List; 041 042@Service 043public class MdmSearchParamSvc { 044 @Autowired 045 FhirContext myFhirContext; 046 047 @Autowired 048 private MatchUrlService myMatchUrlService; 049 050 @Autowired 051 private ISearchParamRegistry mySearchParamRegistry; 052 053 @Autowired 054 private SearchParamExtractorService mySearchParamExtractorService; 055 056 @Autowired 057 private SearchBuilderFactory mySearchBuilderFactory; 058 059 @Autowired 060 private DaoRegistry myDaoRegistry; 061 062 public SearchParameterMap mapFromCriteria(String theResourceType, String theResourceCriteria) { 063 RuntimeResourceDefinition resourceDef = myFhirContext.getResourceDefinition(theResourceType); 064 return myMatchUrlService.translateMatchUrl(theResourceCriteria, resourceDef); 065 } 066 067 public List<String> getValueFromResourceForSearchParam(IBaseResource theResource, String theSearchParam) { 068 String resourceType = myFhirContext.getResourceType(theResource); 069 String searchParam = SearchParameterUtil.stripModifier(theSearchParam); 070 RuntimeSearchParam activeSearchParam = mySearchParamRegistry.getActiveSearchParam( 071 resourceType, searchParam, ISearchParamRegistry.SearchParamLookupContextEnum.SEARCH); 072 return mySearchParamExtractorService.extractParamValuesAsStrings(activeSearchParam, theResource); 073 } 074 075 /** 076 * Given a source type, and a criteria string of the shape {@code name=x&birthDate=y}, generate a {@link SearchParameterMap} 077 * that represents this query. 078 * 079 * @param theSourceType the resource type to execute the search on 080 * @param theCriteria the string search criteria. 081 * @return the generated SearchParameterMap, or an empty one if there is no criteria. 082 */ 083 public SearchParameterMap getSearchParameterMapFromCriteria(String theSourceType, @Nullable String theCriteria) { 084 SearchParameterMap spMap; 085 if (StringUtils.isBlank(theCriteria)) { 086 spMap = new SearchParameterMap(); 087 } else { 088 spMap = mapFromCriteria(theSourceType, theCriteria); 089 } 090 return spMap; 091 } 092 093 public ISearchBuilder generateSearchBuilderForType(String theSourceType) { 094 IFhirResourceDao resourceDao = myDaoRegistry.getResourceDao(theSourceType); 095 return mySearchBuilderFactory.newSearchBuilder(theSourceType, resourceDao.getResourceType()); 096 } 097 098 /** 099 * Will return true if the types match, or the search param type is '*', otherwise false. 100 * 101 * @param theSearchParamType 102 * @param theResourceType 103 * @return 104 */ 105 public boolean searchParamTypeIsValidForResourceType(String theSearchParamType, String theResourceType) { 106 return theSearchParamType.equalsIgnoreCase(theResourceType) || theSearchParamType.equalsIgnoreCase("*"); 107 } 108}