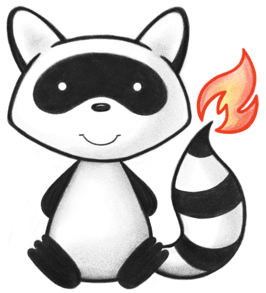
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.util; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.i18n.Msg; 024import ca.uhn.fhir.mdm.model.CanonicalEID; 025import ca.uhn.fhir.rest.server.exceptions.InternalErrorException; 026import ca.uhn.fhir.util.CanonicalIdentifier; 027import org.hl7.fhir.instance.model.api.IBase; 028 029public final class IdentifierUtil { 030 031 private IdentifierUtil() {} 032 033 public static CanonicalIdentifier identifierDtFromIdentifier(IBase theIdentifier) { 034 CanonicalIdentifier retval = new CanonicalIdentifier(); 035 036 // TODO add other fields like "use" etc 037 if (theIdentifier instanceof org.hl7.fhir.dstu3.model.Identifier) { 038 org.hl7.fhir.dstu3.model.Identifier ident = (org.hl7.fhir.dstu3.model.Identifier) theIdentifier; 039 retval.setSystem(ident.getSystem()).setValue(ident.getValue()); 040 } else if (theIdentifier instanceof org.hl7.fhir.r4.model.Identifier) { 041 org.hl7.fhir.r4.model.Identifier ident = (org.hl7.fhir.r4.model.Identifier) theIdentifier; 042 retval.setSystem(ident.getSystem()).setValue(ident.getValue()); 043 } else if (theIdentifier instanceof org.hl7.fhir.r5.model.Identifier) { 044 org.hl7.fhir.r5.model.Identifier ident = (org.hl7.fhir.r5.model.Identifier) theIdentifier; 045 retval.setSystem(ident.getSystem()).setValue(ident.getValue()); 046 } else { 047 throw new InternalErrorException(Msg.code(1486) + "Expected 'Identifier' type but was '" 048 + theIdentifier.getClass().getName() + "'"); 049 } 050 return retval; 051 } 052 053 /** 054 * Retrieves appropriate FHIR Identifier model instance based on the context version 055 * 056 * @param theFhirContext FHIR context to use for determining the identifier version 057 * @param eid EID to get equivalent FHIR Identifier from 058 * @param <T> Generic Identifier base interface 059 * @return Returns appropriate R5, R4 or DSTU3 Identifier instance 060 */ 061 public static <T extends IBase> T toId(FhirContext theFhirContext, CanonicalEID eid) { 062 switch (theFhirContext.getVersion().getVersion()) { 063 case R5: 064 return (T) eid.toR5(); 065 case R4: 066 return (T) eid.toR4(); 067 case DSTU3: 068 return (T) eid.toDSTU3(); 069 } 070 throw new IllegalStateException(Msg.code(1487) + "Unsupported FHIR version " 071 + theFhirContext.getVersion().getVersion()); 072 } 073}