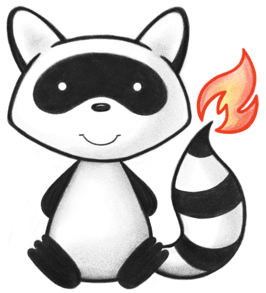
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.util; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.interceptor.model.RequestPartitionId; 024import ca.uhn.fhir.mdm.api.IMdmSettings; 025import ca.uhn.fhir.rest.api.Constants; 026import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 027import org.apache.commons.lang3.StringUtils; 028import org.hl7.fhir.instance.model.api.IAnyResource; 029import org.springframework.stereotype.Service; 030 031@Service 032public class MdmPartitionHelper { 033 private final MessageHelper myMessageHelper; 034 private final IMdmSettings myMdmSettings; 035 036 public MdmPartitionHelper(MessageHelper theMessageHelper, IMdmSettings theMdmSettings) { 037 myMessageHelper = theMessageHelper; 038 myMdmSettings = theMdmSettings; 039 } 040 041 /** 042 * Checks the partition of the two resources are in compliance with the settings 043 * If the mdm settings states mdm resources that only matches against resources in the same partition, validate 044 * the resources have the same partition 045 * This is used to check in merging golden resources as well as when creating a link between source and golden resource 046 * @param theFromResource 047 * @param theToResource 048 */ 049 public void validateMdmResourcesPartitionMatches(IAnyResource theFromResource, IAnyResource theToResource) { 050 if (!myMdmSettings.getSearchAllPartitionForMatch()) { 051 RequestPartitionId fromGoldenResourcePartitionId = 052 (RequestPartitionId) theFromResource.getUserData(Constants.RESOURCE_PARTITION_ID); 053 RequestPartitionId toGoldenPartitionId = 054 (RequestPartitionId) theToResource.getUserData(Constants.RESOURCE_PARTITION_ID); 055 if (fromGoldenResourcePartitionId != null 056 && toGoldenPartitionId != null 057 && fromGoldenResourcePartitionId.hasPartitionIds() 058 && toGoldenPartitionId.hasPartitionIds() 059 && !fromGoldenResourcePartitionId.hasPartitionId(toGoldenPartitionId.getFirstPartitionIdOrNull())) { 060 throw new InvalidRequestException(Msg.code(2075) 061 + myMessageHelper.getMessageForMismatchPartition(theFromResource, theToResource)); 062 } 063 } 064 } 065 066 /** 067 * Generates the request partition id for a mdm candidate search for a given resource. 068 * If the system is configured to search across all partition for matches, this will return all partition 069 * and if not, this function will return the request partition id of the source resource 070 * @param theResource 071 * @return The RequestPartitionId that should be used for the candidate search for the given resource 072 */ 073 public RequestPartitionId getRequestPartitionIdFromResourceForSearch(IAnyResource theResource) { 074 if (myMdmSettings.getSearchAllPartitionForMatch()) { 075 return RequestPartitionId.allPartitions(); 076 } else { 077 return (RequestPartitionId) theResource.getUserData(Constants.RESOURCE_PARTITION_ID); 078 } 079 } 080 081 public RequestPartitionId getRequestPartitionIdForNewGoldenResources(IAnyResource theSourceResource) { 082 if (StringUtils.isBlank(myMdmSettings.getGoldenResourcePartitionName())) { 083 return (RequestPartitionId) theSourceResource.getUserData(Constants.RESOURCE_PARTITION_ID); 084 } else { 085 return RequestPartitionId.fromPartitionName(myMdmSettings.getGoldenResourcePartitionName()); 086 } 087 } 088}