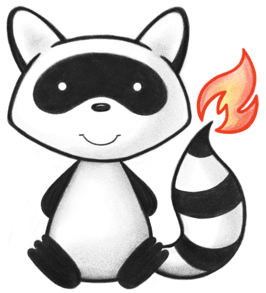
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.util; 021 022import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 023import ca.uhn.fhir.mdm.api.MdmConstants; 024import ca.uhn.fhir.mdm.rules.json.MdmRulesJson; 025import ca.uhn.fhir.rest.param.TokenAndListParam; 026import ca.uhn.fhir.rest.param.TokenParam; 027 028public class MdmSearchParamBuildingUtils { 029 030 private static final String IDENTIFIER = "identifier"; 031 032 private static final String TAG = "_tag"; 033 034 /** 035 * Builds a search parameter map that can be used to find the 036 * golden resources associated with MDM blocked resources (ie, those 037 * resources that were omitted from MDM matching). 038 */ 039 public static SearchParameterMap buildSearchParameterForBlockedResourceCount(String theResourceType) { 040 SearchParameterMap map = new SearchParameterMap(); 041 map.setLoadSynchronous(true); 042 TokenAndListParam tagsToSearch = new TokenAndListParam(); 043 tagsToSearch.addAnd(new TokenParam(MdmConstants.SYSTEM_GOLDEN_RECORD_STATUS, MdmConstants.CODE_GOLDEN_RECORD)); 044 tagsToSearch.addAnd(new TokenParam(MdmConstants.SYSTEM_GOLDEN_RECORD_STATUS, MdmConstants.CODE_BLOCKED)); 045 046 map.add(TAG, tagsToSearch); 047 return map; 048 } 049 050 /** 051 * Creates a SearchParameterMap used for searching for golden resources 052 * by EID specifically. 053 */ 054 public static SearchParameterMap buildEidSearchParameterMap( 055 String theEid, String theResourceType, MdmRulesJson theMdmRules) { 056 SearchParameterMap map = buildBasicGoldenResourceSearchParameterMap(theEid); 057 map.add(IDENTIFIER, new TokenParam(theMdmRules.getEnterpriseEIDSystemForResourceType(theResourceType), theEid)); 058 return map; 059 } 060 061 /** 062 * Creates a SearchParameterMap that can be used to find golden resources. 063 */ 064 public static SearchParameterMap buildBasicGoldenResourceSearchParameterMap(String theResourceType) { 065 SearchParameterMap map = new SearchParameterMap(); 066 map.setLoadSynchronous(true); 067 map.add(TAG, new TokenParam(MdmConstants.SYSTEM_GOLDEN_RECORD_STATUS, MdmConstants.CODE_GOLDEN_RECORD)); 068 return map; 069 } 070}