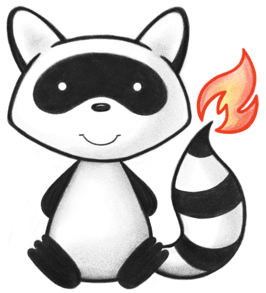
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.util; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.mdm.api.IMdmSettings; 024import ca.uhn.fhir.mdm.api.MdmConstants; 025import ca.uhn.fhir.mdm.api.MdmMatchResultEnum; 026import ca.uhn.fhir.rest.server.provider.ProviderConstants; 027import org.hl7.fhir.instance.model.api.IAnyResource; 028import org.springframework.beans.factory.annotation.Autowired; 029import org.springframework.stereotype.Service; 030 031@Service 032public class MessageHelper { 033 034 @Autowired 035 private final IMdmSettings myMdmSettings; 036 037 @Autowired 038 private final FhirContext myFhirContext; 039 040 public MessageHelper(IMdmSettings theMdmSettings, FhirContext theFhirContext) { 041 myMdmSettings = theMdmSettings; 042 myFhirContext = theFhirContext; 043 } 044 045 public String getMessageForUnmanagedResource() { 046 return String.format( 047 "Only MDM managed resources can be merged. MDM managed resources must have the %s tag.", 048 MdmConstants.CODE_HAPI_MDM_MANAGED); 049 } 050 051 public String getMessageForUnsupportedResource(String theName, IAnyResource theResource) { 052 return getMessageForUnsupportedResource(theName, myFhirContext.getResourceType(theResource)); 053 } 054 055 public String getMessageForUnsupportedResource(String theName, String theResourceType) { 056 return String.format( 057 "Only %s resources can be merged. The %s points to a %s", 058 myMdmSettings.getSupportedMdmTypes(), theName, theResourceType); 059 } 060 061 public String getMessageForUnsupportedMatchResult() { 062 return "Match Result may only be set to " + MdmMatchResultEnum.NO_MATCH + " or " + MdmMatchResultEnum.MATCH; 063 } 064 065 public String getMessageForUnsupportedFirstArgumentTypeInUpdate(String goldenRecordType) { 066 return "First argument to " + ProviderConstants.MDM_UPDATE_LINK + " must be a " 067 + myMdmSettings.getSupportedMdmTypes() + ". Was " + goldenRecordType; 068 } 069 070 public String getMessageForUnsupportedSecondArgumentTypeInUpdate(String theGoldenRecordType) { 071 return "First argument to " + ProviderConstants.MDM_UPDATE_LINK + " must be a " 072 + myMdmSettings.getSupportedMdmTypes() + ". Was " + theGoldenRecordType; 073 } 074 075 public String getMessageForArgumentTypeMismatchInUpdate(String theGoldenRecordType, String theSourceResourceType) { 076 return "Arguments to " + ProviderConstants.MDM_UPDATE_LINK + " must be of the same type. Were " 077 + theGoldenRecordType + " and " + theSourceResourceType; 078 } 079 080 public String getMessageForUnsupportedSourceResource() { 081 return "The source resource is marked with the " + MdmConstants.CODE_NO_MDM_MANAGED 082 + " tag which means it may not be MDM linked."; 083 } 084 085 public String getMessageForNoLink(IAnyResource theGoldenRecord, IAnyResource theSourceResource) { 086 return getMessageForNoLink( 087 theGoldenRecord.getIdElement().toVersionless().toString(), 088 theSourceResource.getIdElement().toVersionless().toString()); 089 } 090 091 public String getMessageForNoLink(String theGoldenRecord, String theSourceResource) { 092 return "No link exists between " + theGoldenRecord + " and " + theSourceResource; 093 } 094 095 public String getMessageForAlreadyAcceptedLink(IAnyResource theGoldenRecord, IAnyResource theSourceResource) { 096 return getMessageForAlreadyAcceptedLink( 097 theGoldenRecord.getIdElement().toVersionless().toString(), 098 theSourceResource.getIdElement().toVersionless().toString()); 099 } 100 101 public String getMessageForAlreadyAcceptedLink(String theGoldenId, String theSourceId) { 102 return "A match with a different golden resource (" + theGoldenId + ") exists for resource " + theSourceId; 103 } 104 105 public String getMessageForPresentLink(IAnyResource theGoldenRecord, IAnyResource theSourceResource) { 106 return getMessageForPresentLink( 107 theGoldenRecord.getIdElement().toVersionless().toString(), 108 theSourceResource.getIdElement().toVersionless().toString()); 109 } 110 111 public String getMessageForPresentLink(String theGoldenRecord, String theSourceResource) { 112 return "Link already exists between " + theGoldenRecord + " and " + theSourceResource 113 + ". Use $mdm-update-link instead."; 114 } 115 116 public String getMessageForMultipleGoldenRecords(IAnyResource theSourceResource) { 117 return getMessageForMultipleGoldenRecords( 118 theSourceResource.getIdElement().toVersionless().toString()); 119 } 120 121 public String getMessageForMultipleGoldenRecords(String theSourceResource) { 122 return theSourceResource + " already has matched golden resource. Use $mdm-query-links to see more details."; 123 } 124 125 public String getMessageForFailedGoldenResourceLoad(String theParamName, String theGoldenResourceId) { 126 return theGoldenResourceId + " used as parameter [" + theParamName 127 + "] could not be loaded as a golden resource, as it appears to be lacking the golden resource meta tags."; 128 } 129 130 public String getMessageForMismatchPartition(IAnyResource theGoldenRecord, IAnyResource theSourceResource) { 131 return getMessageForMismatchPartition( 132 theGoldenRecord.getIdElement().toVersionless().toString(), 133 theSourceResource.getIdElement().toVersionless().toString()); 134 } 135 136 public String getMessageForMismatchPartition(String theGoldenRecord, String theSourceResource) { 137 return theGoldenRecord + " and " + theSourceResource 138 + " are stored in different partitions. This operation is only available for resources on the same partition."; 139 } 140}