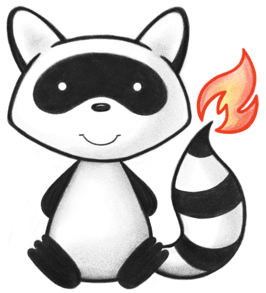
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.util; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.i18n.Msg; 024import org.apache.commons.lang3.StringUtils; 025import org.hl7.fhir.instance.model.api.IBase; 026import org.hl7.fhir.r4.model.HumanName; 027import org.hl7.fhir.r4.model.PrimitiveType; 028 029import java.util.List; 030import java.util.stream.Collectors; 031 032public final class NameUtil { 033 034 private NameUtil() {} 035 036 public static List<String> extractGivenNames(FhirContext theFhirContext, IBase theBase) { 037 switch (theFhirContext.getVersion().getVersion()) { 038 case R4: 039 HumanName humanNameR4 = (HumanName) theBase; 040 return humanNameR4.getGiven().stream() 041 .map(PrimitiveType::getValueAsString) 042 .filter(s -> !StringUtils.isEmpty(s)) 043 .collect(Collectors.toList()); 044 case DSTU3: 045 org.hl7.fhir.dstu3.model.HumanName humanNameDSTU3 = (org.hl7.fhir.dstu3.model.HumanName) theBase; 046 return humanNameDSTU3.getGiven().stream() 047 .map(given -> given.toString()) 048 .filter(s -> !StringUtils.isEmpty(s)) 049 .collect(Collectors.toList()); 050 default: 051 throw new UnsupportedOperationException(Msg.code(1491) + "Version not supported: " 052 + theFhirContext.getVersion().getVersion()); 053 } 054 } 055 056 public static String extractFamilyName(FhirContext theFhirContext, IBase theBase) { 057 switch (theFhirContext.getVersion().getVersion()) { 058 case R4: 059 HumanName humanNameR4 = (HumanName) theBase; 060 return humanNameR4.getFamily(); 061 case DSTU3: 062 org.hl7.fhir.dstu3.model.HumanName humanNameDSTU3 = (org.hl7.fhir.dstu3.model.HumanName) theBase; 063 return humanNameDSTU3.getFamily(); 064 default: 065 throw new UnsupportedOperationException(Msg.code(1492) + "Version not supported: " 066 + theFhirContext.getVersion().getVersion()); 067 } 068 } 069}