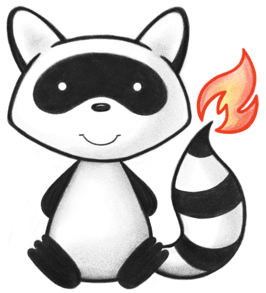
001/*- 002 * #%L 003 * HAPI FHIR - Master Data Management 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.mdm.util; 021 022import org.hl7.fhir.instance.model.api.IPrimitiveType; 023 024import java.lang.reflect.Field; 025import java.util.function.BiPredicate; 026 027public class PrimitiveTypeEqualsPredicate implements BiPredicate { 028 029 @Override 030 public boolean test(Object theBase1, Object theBase2) { 031 if (theBase1 == null) { 032 return theBase2 == null; 033 } 034 if (theBase2 == null) { 035 return false; 036 } 037 if (!theBase1.getClass().equals(theBase2.getClass())) { 038 return false; 039 } 040 041 for (Field f : theBase1.getClass().getDeclaredFields()) { 042 Class<?> fieldClass = f.getType(); 043 044 if (!IPrimitiveType.class.isAssignableFrom(fieldClass)) { 045 continue; 046 } 047 048 IPrimitiveType<?> val1, val2; 049 050 f.setAccessible(true); 051 try { 052 val1 = (IPrimitiveType<?>) f.get(theBase1); 053 val2 = (IPrimitiveType<?>) f.get(theBase2); 054 } catch (Exception e) { 055 // swallow 056 continue; 057 } 058 059 if (val1 == null && val2 == null) { 060 continue; 061 } 062 063 if (val1 == null || val2 == null) { 064 return false; 065 } 066 067 Object actualVal1 = val1.getValue(); 068 Object actualVal2 = val2.getValue(); 069 070 if (actualVal1 == null && actualVal2 == null) { 071 continue; 072 } 073 if (actualVal1 == null) { 074 return false; 075 } 076 if (!actualVal1.equals(actualVal2)) { 077 return false; 078 } 079 } 080 081 return true; 082 } 083}