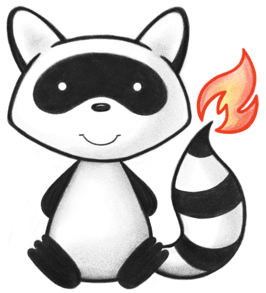
Class TransactionDetails
java.lang.Object
ca.uhn.fhir.rest.api.server.storage.TransactionDetails
This object contains runtime information that is gathered and relevant to a single database transaction.
This doesn't mean a FHIR transaction necessarily, but rather any operation that happens within a single DB transaction
(i.e. a FHIR create, read, transaction, etc.).
The intent with this class is to hold things we want to pass from operation to operation within a transaction in order to avoid looking things up multiple times, etc.
- Since:
- 5.0.0
-
Field Summary
Fields -
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionvoid
addAutoCreatedPlaceholderResource
(org.hl7.fhir.instance.model.api.IIdType theResource) void
addDeferredInterceptorBroadcast
(ca.uhn.fhir.interceptor.api.Pointcut thePointcut, ca.uhn.fhir.interceptor.api.HookParams theHookParams) This can be used by processors for FHIR transactions to defer interceptor broadcasts on sub-requests if neededvoid
addDeletedResourceId
(IResourcePersistentId theResourceId) void
addDeletedResourceIds
(Collection<? extends IResourcePersistentId> theResourceIds) void
addResolvedMatchUrl
(ca.uhn.fhir.context.FhirContext theFhirContext, String theConditionalUrl, IResourcePersistentId<?> thePersistentId) A Resolved Conditional URL is a mapping between a conditional URL (e.gvoid
addResolvedResource
(org.hl7.fhir.instance.model.api.IIdType theResourceId, Supplier<org.hl7.fhir.instance.model.api.IBaseResource> theResource) A Resolved Resource ID is a mapping between a resource ID (e.gvoid
addResolvedResource
(org.hl7.fhir.instance.model.api.IIdType theResourceId, org.hl7.fhir.instance.model.api.IBaseResource theResource) A Resolved Resource ID is a mapping between a resource ID (e.gvoid
addResolvedResourceId
(org.hl7.fhir.instance.model.api.IIdType theResourceId, IResourcePersistentId thePersistentId) A Resolved Resource ID is a mapping between a resource ID (e.gvoid
addRollbackUndoAction
(Runnable theRunnable) Add an action that should be executed if the transaction is rolled backvoid
addUpdatedResourceId
(IResourcePersistentId theResourceId) void
addUpdatedResourceIds
(Collection<? extends IResourcePersistentId> theResourceIds) void
beginAcceptingDeferredInterceptorBroadcasts
(ca.uhn.fhir.interceptor.api.Pointcut... thePointcuts) This can be used by processors for FHIR transactions to defer interceptor broadcasts on sub-requests if neededvoid
void
Clears any previously added rollback actionsvoid
clearUserData
(String theKey) Remove an item previously stored in user datavoid
com.google.common.collect.ListMultimap
<ca.uhn.fhir.interceptor.api.Pointcut, ca.uhn.fhir.interceptor.api.HookParams> This can be used by processors for FHIR transactions to defer interceptor broadcasts on sub-requests if neededList
<org.hl7.fhir.instance.model.api.IIdType> ca.uhn.fhir.rest.api.InterceptorInvocationTimingEnum
getInvocationTiming
(ca.uhn.fhir.interceptor.api.Pointcut thePointcut) <T> T
getOrCreateUserData
(String theKey, Supplier<T> theSupplier) Fetches the existing value in the user data map, or uses theSupplier to create a new object and puts that in the map, and returns itorg.hl7.fhir.instance.model.api.IBaseResource
getResolvedResource
(org.hl7.fhir.instance.model.api.IIdType theId) A Resolved Resource ID is a mapping between a resource ID (e.ggetResolvedResourceId
(org.hl7.fhir.instance.model.api.IIdType theId) A Resolved Resource ID is a mapping between a resource ID (e.gGet the actions that should be executed if the transaction is rolled backThis is the wall-clock time that a given transaction started.<T> T
getUserData
(String theKey) Gets an arbitrary object that will last the lifetime of the current transactionboolean
hasNullResolvedResourceId
(org.hl7.fhir.instance.model.api.IIdType theId) Returns true if the given ID was marked as not existing (i.e. someone calledaddResolvedResourceId(IIdType, IResourcePersistentId)
with an ID of null).boolean
hasResolvedResourceId
(org.hl7.fhir.instance.model.api.IIdType theId) Was the given resource ID resolved previously in this transactionboolean
boolean
This can be used by processors for FHIR transactions to defer interceptor broadcasts on sub-requests if neededboolean
isAcceptingDeferredInterceptorBroadcasts
(ca.uhn.fhir.interceptor.api.Pointcut thePointcut) This can be used by processors for FHIR transactions to defer interceptor broadcasts on sub-requests if neededboolean
boolean
isResolvedResourceIdEmpty
(org.hl7.fhir.instance.model.api.IIdType theId) Was the given resource ID resolved previously in this transaction as not existingvoid
putUserData
(String theKey, Object theValue) Sets an arbitrary object that will last the lifetime of the current transactionvoid
removeResolvedMatchUrl
(String theMatchUrl) void
setFhirTransaction
(boolean theFhirTransaction)
-
Field Details
-
NOT_FOUND
-
-
Constructor Details
-
TransactionDetails
public TransactionDetails()Constructor -
TransactionDetails
Constructor
-
-
Method Details
-
getRollbackUndoActions
Get the actions that should be executed if the transaction is rolled back- Since:
- 5.5.0
-
addRollbackUndoAction
Add an action that should be executed if the transaction is rolled back- Since:
- 5.5.0
-
clearRollbackUndoActions
Clears any previously added rollback actions- Since:
- 5.5.0
-
addUpdatedResourceId
- Since:
- 7.6.0
-
addUpdatedResourceIds
- Since:
- 7.6.0
-
getUpdatedResourceIds
- Since:
- 7.6.0
-
addDeletedResourceId
- Since:
- 6.8.0
-
addDeletedResourceIds
- Since:
- 6.8.0
-
getDeletedResourceIds
- Since:
- 6.8.0
-
getResolvedResourceId
@Nullable public IResourcePersistentId getResolvedResourceId(org.hl7.fhir.instance.model.api.IIdType theId) A Resolved Resource ID is a mapping between a resource ID (e.g. "Patient/ABC
" or "Observation/123
") and a storage ID for that resource. Resources should only be placed within the TransactionDetails if they are known to exist and be valid targets for other resources to link to. -
getResolvedResource
@Nullable public org.hl7.fhir.instance.model.api.IBaseResource getResolvedResource(org.hl7.fhir.instance.model.api.IIdType theId) A Resolved Resource ID is a mapping between a resource ID (e.g. "Patient/ABC
" or "Observation/123
") and the actual persisted/resolved resource with this ID. -
isResolvedResourceIdEmpty
Was the given resource ID resolved previously in this transaction as not existing -
hasResolvedResourceId
Was the given resource ID resolved previously in this transaction -
hasNullResolvedResourceId
Returns true if the given ID was marked as not existing (i.e. someone calledaddResolvedResourceId(IIdType, IResourcePersistentId)
with an ID of null).- Parameters:
theId
- The resource ID- Since:
- 8.0.0
-
addResolvedResourceId
public void addResolvedResourceId(org.hl7.fhir.instance.model.api.IIdType theResourceId, @Nullable IResourcePersistentId thePersistentId) A Resolved Resource ID is a mapping between a resource ID (e.g. "Patient/ABC
" or "Observation/123
") and a storage ID for that resource. Resources should only be placed within the TransactionDetails if they are known to exist and be valid targets for other resources to link to. -
addResolvedResource
public void addResolvedResource(org.hl7.fhir.instance.model.api.IIdType theResourceId, @Nonnull Supplier<org.hl7.fhir.instance.model.api.IBaseResource> theResource) A Resolved Resource ID is a mapping between a resource ID (e.g. "Patient/ABC
" or "Observation/123
") and the actual persisted/resolved resource. This version takes aSupplier
which will only be fetched if the resource is actually needed. This is good in cases where the resource is lazy loaded. -
addResolvedResource
public void addResolvedResource(org.hl7.fhir.instance.model.api.IIdType theResourceId, @Nonnull org.hl7.fhir.instance.model.api.IBaseResource theResource) A Resolved Resource ID is a mapping between a resource ID (e.g. "Patient/ABC
" or "Observation/123
") and the actual persisted/resolved resource. -
getResolvedMatchUrls
-
addResolvedMatchUrl
public void addResolvedMatchUrl(ca.uhn.fhir.context.FhirContext theFhirContext, String theConditionalUrl, @Nonnull IResourcePersistentId<?> thePersistentId) A Resolved Conditional URL is a mapping between a conditional URL (e.g. "Patient?identifier=foo|bar
" or "Observation/123
") and a storage ID for that resource. Resources should only be placed within the TransactionDetails if they are known to exist and be valid targets for other resources to link to. -
removeResolvedMatchUrl
- Since:
- 6.8.0
- See Also:
-
getTransactionDate
This is the wall-clock time that a given transaction started. -
clearUserData
Remove an item previously stored in user data- See Also:
-
putUserData
Sets an arbitrary object that will last the lifetime of the current transaction- See Also:
-
getUserData
Gets an arbitrary object that will last the lifetime of the current transaction- See Also:
-
getOrCreateUserData
Fetches the existing value in the user data map, or uses theSupplier to create a new object and puts that in the map, and returns it -
beginAcceptingDeferredInterceptorBroadcasts
public void beginAcceptingDeferredInterceptorBroadcasts(ca.uhn.fhir.interceptor.api.Pointcut... thePointcuts) This can be used by processors for FHIR transactions to defer interceptor broadcasts on sub-requests if needed- Since:
- 5.2.0
-
isAcceptingDeferredInterceptorBroadcasts
This can be used by processors for FHIR transactions to defer interceptor broadcasts on sub-requests if needed- Since:
- 5.2.0
-
isAcceptingDeferredInterceptorBroadcasts
public boolean isAcceptingDeferredInterceptorBroadcasts(ca.uhn.fhir.interceptor.api.Pointcut thePointcut) This can be used by processors for FHIR transactions to defer interceptor broadcasts on sub-requests if needed- Since:
- 5.2.0
-
endAcceptingDeferredInterceptorBroadcasts
public com.google.common.collect.ListMultimap<ca.uhn.fhir.interceptor.api.Pointcut,ca.uhn.fhir.interceptor.api.HookParams> endAcceptingDeferredInterceptorBroadcasts()This can be used by processors for FHIR transactions to defer interceptor broadcasts on sub-requests if needed- Since:
- 5.2.0
-
addDeferredInterceptorBroadcast
public void addDeferredInterceptorBroadcast(ca.uhn.fhir.interceptor.api.Pointcut thePointcut, ca.uhn.fhir.interceptor.api.HookParams theHookParams) This can be used by processors for FHIR transactions to defer interceptor broadcasts on sub-requests if needed- Since:
- 5.2.0
-
getInvocationTiming
public ca.uhn.fhir.rest.api.InterceptorInvocationTimingEnum getInvocationTiming(ca.uhn.fhir.interceptor.api.Pointcut thePointcut) -
deferredBroadcastProcessingFinished
-
clearResolvedItems
-
hasResolvedResourceIds
-
isFhirTransaction
-
setFhirTransaction
-
addAutoCreatedPlaceholderResource
-
getAutoCreatedPlaceholderResourcesAndClear
@Nonnull public List<org.hl7.fhir.instance.model.api.IIdType> getAutoCreatedPlaceholderResourcesAndClear()
-