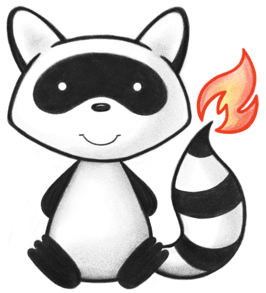
Class CorsInterceptor
java.lang.Object
ca.uhn.fhir.rest.server.interceptor.InterceptorAdapter
ca.uhn.fhir.rest.server.interceptor.CorsInterceptor
- All Implemented Interfaces:
IServerInterceptor
-
Constructor Summary
ConstructorsConstructorDescriptionConstructor which creates an interceptor with default CORS configuration for use in a FHIR server.CorsInterceptor
(org.springframework.web.cors.CorsConfiguration theConfiguration) Constructor which accepts the given configuration -
Method Summary
Modifier and TypeMethodDescriptionorg.springframework.web.cors.CorsConfiguration
Gets the CORS configurationboolean
incomingRequestPreProcessed
(jakarta.servlet.http.HttpServletRequest theRequest, jakarta.servlet.http.HttpServletResponse theResponse) This method is called before any other processing takes place for each incoming request.void
setConfig
(org.springframework.web.cors.CorsConfiguration theConfiguration) Sets the CORS configurationMethods inherited from class ca.uhn.fhir.rest.server.interceptor.InterceptorAdapter
handleException, incomingRequestPostProcessed, incomingRequestPreHandled, outgoingResponse, outgoingResponse, outgoingResponse, outgoingResponse, outgoingResponse, outgoingResponse, outgoingResponse, preProcessOutgoingException, processingCompletedNormally
-
Constructor Details
-
CorsInterceptor
public CorsInterceptor()Constructor which creates an interceptor with default CORS configuration for use in a FHIR server. This includes:- Allowed Origin: *
- Allowed Header: Accept
- Allowed Header: Access-Control-Request-Headers
- Allowed Header: Access-Control-Request-Method
- Allowed Header: Cache-Control
- Exposed Header: Content-Location
- Allowed Header: Content-Type
- Exposed Header: Location
- Allowed Header: Origin
- Allowed Header: Prefer
- Allowed Header: X-Requested-With
-
CorsInterceptor
Constructor which accepts the given configuration- Parameters:
theConfiguration
- The CORS configuration
-
-
Method Details
-
getConfig
Gets the CORS configuration -
setConfig
Sets the CORS configuration -
incomingRequestPreProcessed
public boolean incomingRequestPreProcessed(jakarta.servlet.http.HttpServletRequest theRequest, jakarta.servlet.http.HttpServletResponse theResponse) Description copied from interface:IServerInterceptor
This method is called before any other processing takes place for each incoming request. It may be used to provide alternate handling for some requests, or to screen requests before they are handled, etc.Note that any exceptions thrown by this method will not be trapped by HAPI (they will be passed up to the server)
- Specified by:
incomingRequestPreProcessed
in interfaceIServerInterceptor
- Overrides:
incomingRequestPreProcessed
in classInterceptorAdapter
- Parameters:
theRequest
- The incoming requesttheResponse
- The response. Note that interceptors may choose to provide a response (i.e. by callingServletResponse.getWriter()
) but in that case it is important to returnfalse
to indicate that the server itself should not also provide a response.- Returns:
- Return
true
if processing should continue normally. This is generally the right thing to do. If your interceptor is providing a response rather than letting HAPI handle the response normally, you must returnfalse
. In this case, no further processing will occur and no further interceptors will be called.
-