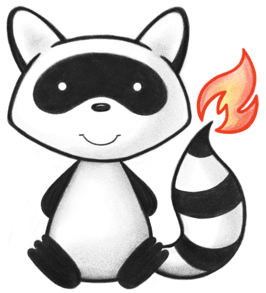
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.api.server; 021 022import org.hl7.fhir.instance.model.api.IBaseResource; 023 024import java.util.Collections; 025import java.util.List; 026 027public class SimplePreResourceAccessDetails implements IPreResourceAccessDetails { 028 029 private final List<IBaseResource> myResources; 030 private final boolean[] myBlocked; 031 032 public SimplePreResourceAccessDetails(IBaseResource theResource) { 033 this(Collections.singletonList(theResource)); 034 } 035 036 public <T extends IBaseResource> SimplePreResourceAccessDetails(List<T> theResources) { 037 //noinspection unchecked 038 myResources = (List<IBaseResource>) theResources; 039 myBlocked = new boolean[myResources.size()]; 040 } 041 042 @Override 043 public int size() { 044 return myResources.size(); 045 } 046 047 @Override 048 public IBaseResource getResource(int theIndex) { 049 return myResources.get(theIndex); 050 } 051 052 @Override 053 public void setDontReturnResourceAtIndex(int theIndex) { 054 myBlocked[theIndex] = true; 055 } 056 057 public boolean isDontReturnResourceAtIndex(int theIndex) { 058 return myBlocked[theIndex]; 059 } 060 061 /** 062 * Remove any blocked resources from the list that was passed into the constructor 063 */ 064 public void applyFilterToList() { 065 for (int i = size() - 1; i >= 0; i--) { 066 if (isDontReturnResourceAtIndex(i)) { 067 myResources.remove(i); 068 } 069 } 070 } 071}