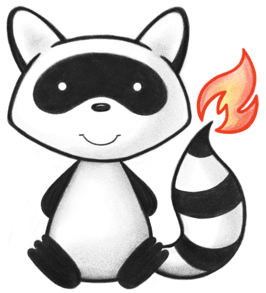
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.api.server; 021 022import com.google.common.collect.Lists; 023import org.apache.commons.lang3.Validate; 024import org.hl7.fhir.instance.model.api.IBaseResource; 025 026import java.util.ArrayList; 027import java.util.Arrays; 028import java.util.Collection; 029import java.util.Collections; 030import java.util.Iterator; 031import java.util.List; 032import java.util.Objects; 033 034public class SimplePreResourceShowDetails implements IPreResourceShowDetails { 035 036 private static final IBaseResource[] EMPTY_RESOURCE_ARRAY = new IBaseResource[0]; 037 038 private final IBaseResource[] myResources; 039 private final boolean[] myResourceMarkedAsSubset; 040 041 /** 042 * Constructor for a single resource 043 */ 044 public SimplePreResourceShowDetails(IBaseResource theResource) { 045 this(Lists.newArrayList(theResource)); 046 } 047 048 /** 049 * Constructor for a collection of resources 050 */ 051 public <T extends IBaseResource> SimplePreResourceShowDetails(Collection<T> theResources) { 052 myResources = theResources.toArray(EMPTY_RESOURCE_ARRAY); 053 myResourceMarkedAsSubset = new boolean[myResources.length]; 054 } 055 056 @Override 057 public int size() { 058 return myResources.length; 059 } 060 061 @Override 062 public IBaseResource getResource(int theIndex) { 063 return myResources[theIndex]; 064 } 065 066 @Override 067 public void setResource(int theIndex, IBaseResource theResource) { 068 Validate.isTrue(theIndex >= 0, "Invalid index %d - theIndex must not be < 0", theIndex); 069 Validate.isTrue( 070 theIndex < myResources.length, 071 "Invalid index {} - theIndex must be < %d", 072 theIndex, 073 myResources.length); 074 myResources[theIndex] = theResource; 075 } 076 077 @Override 078 public void markResourceAtIndexAsSubset(int theIndex) { 079 Validate.isTrue(theIndex >= 0, "Invalid index %d - theIndex must not be < 0", theIndex); 080 Validate.isTrue( 081 theIndex < myResources.length, 082 "Invalid index {} - theIndex must be < %d", 083 theIndex, 084 myResources.length); 085 myResourceMarkedAsSubset[theIndex] = true; 086 } 087 088 @Override 089 public List<IBaseResource> getAllResources() { 090 ArrayList<IBaseResource> retVal = new ArrayList<>(myResources.length); 091 Arrays.stream(myResources).filter(Objects::nonNull).forEach(retVal::add); 092 return Collections.unmodifiableList(retVal); 093 } 094 095 @Override 096 public Iterator<IBaseResource> iterator() { 097 return Arrays.asList(myResources).iterator(); 098 } 099 100 public List<IBaseResource> toList() { 101 return Lists.newArrayList(myResources); 102 } 103}