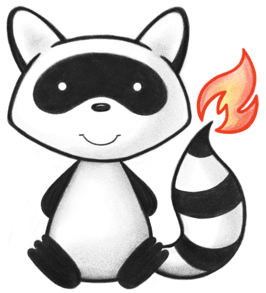
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.api.server.bulk; 021 022import ca.uhn.fhir.interceptor.model.RequestPartitionId; 023import ca.uhn.fhir.model.api.BaseBatchJobParameters; 024import ca.uhn.fhir.rest.server.util.JsonDateDeserializer; 025import ca.uhn.fhir.rest.server.util.JsonDateSerializer; 026import com.fasterxml.jackson.annotation.JsonProperty; 027import com.fasterxml.jackson.databind.annotation.JsonDeserialize; 028import com.fasterxml.jackson.databind.annotation.JsonSerialize; 029 030import java.util.ArrayList; 031import java.util.Collection; 032import java.util.Date; 033import java.util.List; 034 035public class BulkExportJobParameters extends BaseBatchJobParameters { 036 037 /** 038 * List of resource types to export. 039 */ 040 @JsonProperty("resourceTypes") 041 private List<String> myResourceTypes; 042 043 /** 044 * The start date from when we should start 045 * doing the export. (end date is assumed to be "now") 046 */ 047 @JsonSerialize(using = JsonDateSerializer.class) 048 @JsonDeserialize(using = JsonDateDeserializer.class) 049 @JsonProperty("since") 050 private Date mySince; 051 052 @JsonProperty("exportId") 053 private String myExportId; 054 055 /** 056 * Filters are used to narrow down the resources to export. 057 * Eg: 058 * Patient/123?group=a 059 * "group=a" is a filter 060 */ 061 @JsonProperty("filters") 062 private List<String> myFilters; 063 064 /** 065 * URLs to be applied by the inMemoryMatcher after the SQL select 066 */ 067 @JsonProperty("postFetchFilterUrls") 068 private List<String> myPostFetchFilterUrls; 069 070 /** 071 * Output format. 072 * Currently unsupported (all outputs are ndjson) 073 */ 074 @JsonProperty("outputFormat") 075 private String myOutputFormat; 076 077 /** 078 * Export style - Patient, Group or Everything 079 */ 080 @JsonProperty("exportStyle") 081 private ExportStyle myExportStyle; 082 083 /** 084 * Patient id(s) 085 */ 086 @JsonProperty("patientIds") 087 private List<String> myPatientIds = new ArrayList<>(); 088 089 /** 090 * The request which originated the request. 091 */ 092 @JsonProperty("originalRequestUrl") 093 private String myOriginalRequestUrl; 094 095 /** 096 * The group id 097 */ 098 @JsonProperty("groupId") 099 private String myGroupId; 100 101 /** 102 * For group export; 103 * whether or not to expand mdm 104 */ 105 @JsonProperty("expandMdm") 106 private boolean myExpandMdm; 107 108 /** 109 * The partition for the request if applicable. 110 */ 111 @JsonProperty("partitionId") 112 private RequestPartitionId myPartitionId; 113 114 @JsonProperty("binarySecurityContextIdentifierSystem") 115 private String myBinarySecurityContextIdentifierSystem; 116 117 @JsonProperty("binarySecurityContextIdentifierValue") 118 private String myBinarySecurityContextIdentifierValue; 119 120 public String getExportIdentifier() { 121 return myExportId; 122 } 123 124 public void setExportIdentifier(String theExportId) { 125 myExportId = theExportId; 126 } 127 128 public List<String> getResourceTypes() { 129 if (myResourceTypes == null) { 130 myResourceTypes = new ArrayList<>(); 131 } 132 return myResourceTypes; 133 } 134 135 public void setResourceTypes(Collection<String> theResourceTypes) { 136 getResourceTypes().clear(); 137 if (theResourceTypes != null) { 138 getResourceTypes().addAll(theResourceTypes); 139 } 140 } 141 142 public Date getSince() { 143 return mySince; 144 } 145 146 public void setSince(Date theSince) { 147 mySince = theSince; 148 } 149 150 public List<String> getFilters() { 151 if (myFilters == null) { 152 myFilters = new ArrayList<>(); 153 } 154 return myFilters; 155 } 156 157 public void setFilters(Collection<String> theFilters) { 158 getFilters().clear(); 159 if (theFilters != null) { 160 getFilters().addAll(theFilters); 161 } 162 } 163 164 public List<String> getPostFetchFilterUrls() { 165 if (myPostFetchFilterUrls == null) { 166 myPostFetchFilterUrls = new ArrayList<>(); 167 } 168 return myPostFetchFilterUrls; 169 } 170 171 public void setPostFetchFilterUrls(Collection<String> thePostFetchFilterUrls) { 172 getPostFetchFilterUrls().clear(); 173 if (thePostFetchFilterUrls != null) { 174 getPostFetchFilterUrls().addAll(thePostFetchFilterUrls); 175 } 176 } 177 178 public String getOutputFormat() { 179 return myOutputFormat; 180 } 181 182 public void setOutputFormat(String theOutputFormat) { 183 myOutputFormat = theOutputFormat; 184 } 185 186 public ExportStyle getExportStyle() { 187 return myExportStyle; 188 } 189 190 public void setExportStyle(ExportStyle theExportStyle) { 191 myExportStyle = theExportStyle; 192 } 193 194 public List<String> getPatientIds() { 195 if (myPatientIds == null) { 196 myPatientIds = new ArrayList<>(); 197 } 198 return myPatientIds; 199 } 200 201 public void setPatientIds(Collection<String> thePatientIds) { 202 getPatientIds().clear(); 203 if (thePatientIds != null) { 204 getPatientIds().addAll(thePatientIds); 205 } 206 } 207 208 public String getGroupId() { 209 return myGroupId; 210 } 211 212 public void setGroupId(String theGroupId) { 213 myGroupId = theGroupId; 214 } 215 216 public boolean isExpandMdm() { 217 return myExpandMdm; 218 } 219 220 public void setExpandMdm(boolean theExpandMdm) { 221 myExpandMdm = theExpandMdm; 222 } 223 224 public String getOriginalRequestUrl() { 225 return myOriginalRequestUrl; 226 } 227 228 public void setOriginalRequestUrl(String theOriginalRequestUrl) { 229 this.myOriginalRequestUrl = theOriginalRequestUrl; 230 } 231 232 public RequestPartitionId getPartitionId() { 233 return myPartitionId; 234 } 235 236 public void setPartitionId(RequestPartitionId thePartitionId) { 237 this.myPartitionId = thePartitionId; 238 } 239 240 /** 241 * Sets a value to place in the generated Binary resource's 242 * Binary.securityContext.identifier 243 */ 244 public void setBinarySecurityContextIdentifierSystem(String theBinarySecurityContextIdentifierSystem) { 245 myBinarySecurityContextIdentifierSystem = theBinarySecurityContextIdentifierSystem; 246 } 247 248 /** 249 * Sets a value to place in the generated Binary resource's 250 * Binary.securityContext.identifier 251 */ 252 public String getBinarySecurityContextIdentifierSystem() { 253 return myBinarySecurityContextIdentifierSystem; 254 } 255 256 /** 257 * Sets a value to place in the generated Binary resource's 258 * Binary.securityContext.identifier 259 */ 260 public void setBinarySecurityContextIdentifierValue(String theBinarySecurityContextIdentifierValue) { 261 myBinarySecurityContextIdentifierValue = theBinarySecurityContextIdentifierValue; 262 } 263 264 /** 265 * Sets a value to place in the generated Binary resource's 266 * Binary.securityContext.identifier 267 */ 268 public String getBinarySecurityContextIdentifierValue() { 269 return myBinarySecurityContextIdentifierValue; 270 } 271 272 public enum ExportStyle { 273 PATIENT, 274 GROUP, 275 SYSTEM 276 } 277}