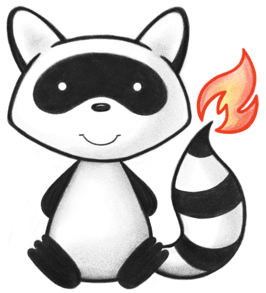
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.api.server.cdshooks; 021 022import com.fasterxml.jackson.annotation.JsonProperty; 023 024/** 025 * A structure holding an OAuth 2.0 bearer access token granting the CDS Service access to FHIR resource 026 */ 027public class CdsServiceRequestAuthorizationJson extends BaseCdsServiceJson { 028 @JsonProperty(value = "access_token", required = true) 029 String myAccessToken; 030 031 @JsonProperty(value = "token_type", required = true) 032 String myTokenType; 033 034 @JsonProperty(value = "expires_in", required = true) 035 Long myExpiresIn; 036 037 @JsonProperty(value = "scope", required = true) 038 String myScope; 039 040 @JsonProperty(value = "subject", required = true) 041 String mySubject; 042 043 public String getAccessToken() { 044 return myAccessToken; 045 } 046 047 public CdsServiceRequestAuthorizationJson setAccessToken(String theAccessToken) { 048 myAccessToken = theAccessToken; 049 return this; 050 } 051 052 public String getTokenType() { 053 return myTokenType; 054 } 055 056 public CdsServiceRequestAuthorizationJson setTokenType(String theTokenType) { 057 myTokenType = theTokenType; 058 return this; 059 } 060 061 public Long getExpiresIn() { 062 return myExpiresIn; 063 } 064 065 public CdsServiceRequestAuthorizationJson setExpiresIn(Long theExpiresIn) { 066 myExpiresIn = theExpiresIn; 067 return this; 068 } 069 070 public String getScope() { 071 return myScope; 072 } 073 074 public CdsServiceRequestAuthorizationJson setScope(String theScope) { 075 myScope = theScope; 076 return this; 077 } 078 079 public String getSubject() { 080 return mySubject; 081 } 082 083 public CdsServiceRequestAuthorizationJson setSubject(String theSubject) { 084 mySubject = theSubject; 085 return this; 086 } 087}