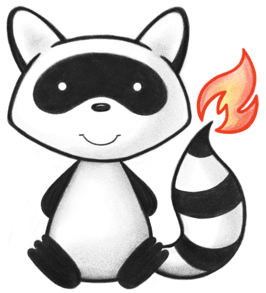
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.api.server.cdshooks; 021 022import com.fasterxml.jackson.annotation.JsonAnyGetter; 023import com.fasterxml.jackson.annotation.JsonIgnoreProperties; 024import org.hl7.fhir.instance.model.api.IBaseResource; 025 026import java.util.Collections; 027import java.util.LinkedHashMap; 028import java.util.List; 029import java.util.Map; 030import java.util.Set; 031 032@JsonIgnoreProperties(ignoreUnknown = true) 033public class CdsServiceRequestContextJson extends BaseCdsServiceJson { 034 035 @JsonAnyGetter 036 private Map<String, Object> myMap; 037 038 public String getString(String theKey) { 039 if (myMap == null) { 040 return null; 041 } 042 return (String) myMap.get(theKey); 043 } 044 045 public List<String> getArray(String theKey) { 046 if (myMap == null) { 047 return Collections.emptyList(); 048 } 049 return (List<String>) myMap.get(theKey); 050 } 051 052 public IBaseResource getResource(String theKey) { 053 if (myMap == null) { 054 return null; 055 } 056 return (IBaseResource) myMap.get(theKey); 057 } 058 059 public void put(String theKey, Object theValue) { 060 if (myMap == null) { 061 myMap = new LinkedHashMap<>(); 062 } 063 myMap.put(theKey, theValue); 064 } 065 066 public Set<String> getKeys() { 067 if (myMap == null) { 068 return Collections.emptySet(); 069 } 070 return myMap.keySet(); 071 } 072 073 public Object get(String theKey) { 074 if (myMap == null) { 075 return null; 076 } 077 return myMap.get(theKey); 078 } 079 080 public boolean containsKey(String theKey) { 081 if (myMap == null) { 082 return false; 083 } 084 return myMap.containsKey(theKey); 085 } 086}