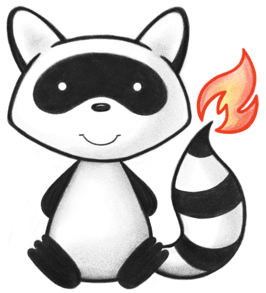
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.api.server.cdshooks; 021 022import com.fasterxml.jackson.annotation.JsonProperty; 023import jakarta.annotation.Nonnull; 024import org.hl7.fhir.instance.model.api.IBaseResource; 025 026import java.util.Collections; 027import java.util.HashSet; 028import java.util.LinkedHashMap; 029import java.util.Map; 030import java.util.Set; 031 032/** 033 * Represents a CDS Hooks Service Request 034 * 035 * @see <a href="https://cds-hooks.hl7.org/ballots/2020Sep/">Version 1.1 of the CDS Hooks Specification</a> 036 */ 037public class CdsServiceRequestJson extends BaseCdsServiceJson { 038 @JsonProperty(value = "hook", required = true) 039 String myHook; 040 041 @JsonProperty(value = "hookInstance", required = true) 042 String myHookInstance; 043 044 @JsonProperty("fhirServer") 045 String myFhirServer; 046 047 @JsonProperty("fhirAuthorization") 048 CdsServiceRequestAuthorizationJson myServiceRequestAuthorizationJson; 049 050 @JsonProperty(value = "context", required = true) 051 CdsServiceRequestContextJson myContext; 052 053 @JsonProperty("prefetch") 054 Map<String, IBaseResource> myPrefetch; 055 056 public String getHookInstance() { 057 return myHookInstance; 058 } 059 060 public CdsServiceRequestJson setHookInstance(String theHookInstance) { 061 myHookInstance = theHookInstance; 062 return this; 063 } 064 065 public String getFhirServer() { 066 return myFhirServer; 067 } 068 069 public CdsServiceRequestJson setFhirServer(String theFhirServer) { 070 myFhirServer = theFhirServer; 071 return this; 072 } 073 074 public String getHook() { 075 return myHook; 076 } 077 078 public CdsServiceRequestJson setHook(String theHook) { 079 myHook = theHook; 080 return this; 081 } 082 083 public CdsServiceRequestContextJson getContext() { 084 if (myContext == null) { 085 myContext = new CdsServiceRequestContextJson(); 086 } 087 return myContext; 088 } 089 090 public CdsServiceRequestJson setContext(CdsServiceRequestContextJson theContext) { 091 myContext = theContext; 092 return this; 093 } 094 095 public CdsServiceRequestAuthorizationJson getServiceRequestAuthorizationJson() { 096 if (myServiceRequestAuthorizationJson == null) { 097 myServiceRequestAuthorizationJson = new CdsServiceRequestAuthorizationJson(); 098 } 099 return myServiceRequestAuthorizationJson; 100 } 101 102 public CdsServiceRequestJson setServiceRequestAuthorizationJson( 103 CdsServiceRequestAuthorizationJson theServiceRequestAuthorizationJson) { 104 myServiceRequestAuthorizationJson = theServiceRequestAuthorizationJson; 105 return this; 106 } 107 108 public void addPrefetch(String theKey, IBaseResource theResource) { 109 if (myPrefetch == null) { 110 myPrefetch = new LinkedHashMap<>(); 111 } 112 myPrefetch.put(theKey, theResource); 113 } 114 115 public IBaseResource getPrefetch(String theKey) { 116 if (myPrefetch == null) { 117 return null; 118 } 119 return myPrefetch.get(theKey); 120 } 121 122 public void removePrefetch(String theKey) { 123 if (myPrefetch == null) { 124 return; 125 } 126 myPrefetch.remove(theKey); 127 } 128 129 public void addContext(String theKey, Object theValue) { 130 getContext().put(theKey, theValue); 131 } 132 133 @Nonnull 134 public Set<String> getPrefetchKeys() { 135 if (myPrefetch == null) { 136 return new HashSet<>(); 137 } 138 return Collections.unmodifiableSet(myPrefetch.keySet()); 139 } 140}