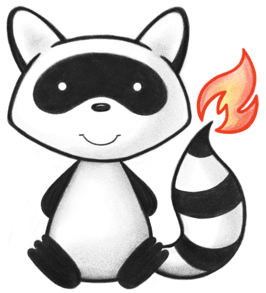
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.api.server.storage; 021 022import org.hl7.fhir.instance.model.api.IIdType; 023 024import java.util.Objects; 025 026/** 027 * This class is an abstraction for however primary keys are stored in the underlying storage engine. This might be 028 * a Long, a String, or something else. The generic type T represents the primary key type. 029 */ 030public abstract class BaseResourcePersistentId<T> implements IResourcePersistentId<T> { 031 private Long myVersion; 032 private final String myResourceType; 033 private IIdType myAssociatedResourceId; 034 035 protected BaseResourcePersistentId(String theResourceType) { 036 myResourceType = theResourceType; 037 } 038 039 protected BaseResourcePersistentId(Long theVersion, String theResourceType) { 040 myVersion = theVersion; 041 myResourceType = theResourceType; 042 } 043 044 @Override 045 public IIdType getAssociatedResourceId() { 046 return myAssociatedResourceId; 047 } 048 049 @Override 050 public IResourcePersistentId<T> setAssociatedResourceId(IIdType theAssociatedResourceId) { 051 myAssociatedResourceId = theAssociatedResourceId; 052 return this; 053 } 054 055 @Override 056 public boolean equals(Object theO) { 057 if (this == theO) return true; 058 if (theO == null || getClass() != theO.getClass()) return false; 059 BaseResourcePersistentId<?> that = (BaseResourcePersistentId<?>) theO; 060 return Objects.equals(myVersion, that.myVersion); 061 } 062 063 @Override 064 public int hashCode() { 065 return Objects.hash(myVersion); 066 } 067 068 @Override 069 public Long getVersion() { 070 return myVersion; 071 } 072 073 /** 074 * @param theVersion This should only be populated if a specific version is needed. If you want the current version, 075 * leave this as <code>null</code> 076 */ 077 @Override 078 public void setVersion(Long theVersion) { 079 myVersion = theVersion; 080 } 081 082 @Override 083 public String getResourceType() { 084 return myResourceType; 085 } 086}