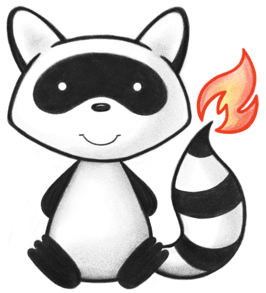
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server; 021 022import ca.uhn.fhir.rest.server.method.OperationMethodBinding; 023import ca.uhn.fhir.rest.server.method.SearchMethodBinding; 024 025import java.util.HashMap; 026import java.util.IdentityHashMap; 027import java.util.List; 028 029public class Bindings { 030 private final IdentityHashMap<SearchMethodBinding, String> myNamedSearchMethodBindingToName; 031 private final HashMap<String, List<SearchMethodBinding>> mySearchNameToBindings; 032 private final HashMap<String, List<OperationMethodBinding>> myOperationIdToBindings; 033 private final IdentityHashMap<OperationMethodBinding, String> myOperationBindingToId; 034 035 public Bindings( 036 IdentityHashMap<SearchMethodBinding, String> theNamedSearchMethodBindingToName, 037 HashMap<String, List<SearchMethodBinding>> theSearchNameToBindings, 038 HashMap<String, List<OperationMethodBinding>> theOperationIdToBindings, 039 IdentityHashMap<OperationMethodBinding, String> theOperationBindingToName) { 040 myNamedSearchMethodBindingToName = theNamedSearchMethodBindingToName; 041 mySearchNameToBindings = theSearchNameToBindings; 042 myOperationIdToBindings = theOperationIdToBindings; 043 myOperationBindingToId = theOperationBindingToName; 044 } 045 046 public IdentityHashMap<SearchMethodBinding, String> getNamedSearchMethodBindingToName() { 047 return myNamedSearchMethodBindingToName; 048 } 049 050 public HashMap<String, List<SearchMethodBinding>> getSearchNameToBindings() { 051 return mySearchNameToBindings; 052 } 053 054 public HashMap<String, List<OperationMethodBinding>> getOperationIdToBindings() { 055 return myOperationIdToBindings; 056 } 057 058 public IdentityHashMap<OperationMethodBinding, String> getOperationBindingToId() { 059 return myOperationBindingToId; 060 } 061}