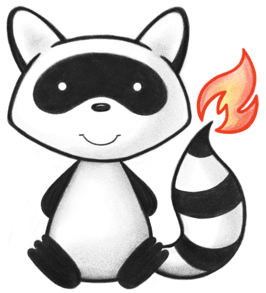
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server; 021 022import ca.uhn.fhir.rest.server.method.ResponsePage; 023import jakarta.annotation.Nonnull; 024import org.apache.commons.lang3.Validate; 025import org.hl7.fhir.instance.model.api.IBaseResource; 026 027import java.util.List; 028 029/** 030 * Bundle provider that uses named pages instead of counts 031 */ 032public class BundleProviderWithNamedPages extends SimpleBundleProvider { 033 034 private String myNextPageId; 035 private String myCurrentPageId; 036 private String myPreviousPageId; 037 038 /** 039 * Constructor 040 * 041 * @param theResultsInThisPage The complete list of results in the current page. Must not be null. 042 * @param theSearchId The ID for the search. Note that you should also populate {@link #setNextPageId(String)} and {@link #setPreviousPageId(String)} if these are known. Must not be <code>null</code> or blank. 043 * @param thePageId The ID for the current page. Note that you should also populate {@link #setNextPageId(String)} and {@link #setPreviousPageId(String)} if these are known. Must not be <code>null</code> or blank. 044 * @param theTotalResults The total number of result (if this is known), or <code>null</code> 045 * @see #setNextPageId(String) 046 * @see #setPreviousPageId(String) 047 */ 048 public BundleProviderWithNamedPages( 049 List<IBaseResource> theResultsInThisPage, String theSearchId, String thePageId, Integer theTotalResults) { 050 super(theResultsInThisPage, theSearchId); 051 052 Validate.notNull(theResultsInThisPage, "theResultsInThisPage must not be null"); 053 Validate.notBlank(thePageId, "thePageId must not be null or blank"); 054 055 setCurrentPageId(thePageId); 056 setSize(theTotalResults); 057 } 058 059 @Override 060 public String getCurrentPageId() { 061 return myCurrentPageId; 062 } 063 064 public BundleProviderWithNamedPages setCurrentPageId(String theCurrentPageId) { 065 myCurrentPageId = theCurrentPageId; 066 return this; 067 } 068 069 @Override 070 public String getNextPageId() { 071 return myNextPageId; 072 } 073 074 public BundleProviderWithNamedPages setNextPageId(String theNextPageId) { 075 myNextPageId = theNextPageId; 076 return this; 077 } 078 079 @Override 080 public String getPreviousPageId() { 081 return myPreviousPageId; 082 } 083 084 public BundleProviderWithNamedPages setPreviousPageId(String thePreviousPageId) { 085 myPreviousPageId = thePreviousPageId; 086 return this; 087 } 088 089 @SuppressWarnings("unchecked") 090 @Nonnull 091 @Override 092 public List<IBaseResource> getResources( 093 int theFromIndex, int theToIndex, @Nonnull ResponsePage.ResponsePageBuilder theResponsePageBuilder) { 094 return (List<IBaseResource>) getList(); // indexes are ignored for this provider type 095 } 096 097 @Override 098 public BundleProviderWithNamedPages setSize(Integer theSize) { 099 super.setSize(theSize); 100 return this; 101 } 102}