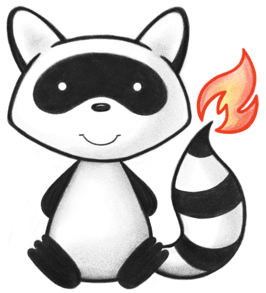
001/* 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server; 021 022import ca.uhn.fhir.model.primitive.InstantDt; 023import ca.uhn.fhir.rest.api.server.IBundleProvider; 024import ca.uhn.fhir.rest.server.method.ResponsePage; 025import ca.uhn.fhir.util.CoverageIgnore; 026import jakarta.annotation.Nonnull; 027import org.hl7.fhir.instance.model.api.IBaseResource; 028 029import java.util.Collections; 030import java.util.List; 031 032/** 033 * Utility methods for working with {@link IBundleProvider} 034 */ 035public class BundleProviders { 036 037 /** Non instantiable */ 038 @CoverageIgnore 039 private BundleProviders() { 040 // nothing 041 } 042 043 /** 044 * Create a new unmodifiable empty resource list with the current time as the publish date. 045 */ 046 public static IBundleProvider newEmptyList() { 047 final InstantDt published = InstantDt.withCurrentTime(); 048 return new IBundleProvider() { 049 @Nonnull 050 @Override 051 public List<IBaseResource> getResources( 052 int theFromIndex, int theToIndex, ResponsePage.ResponsePageBuilder theResponsePageBuilder) { 053 return Collections.emptyList(); 054 } 055 056 @Override 057 public Integer size() { 058 return 0; 059 } 060 061 @Override 062 public InstantDt getPublished() { 063 return published; 064 } 065 066 @Override 067 public Integer preferredPageSize() { 068 return null; 069 } 070 071 @Override 072 public String getUuid() { 073 return null; 074 } 075 }; 076 } 077 078 public static IBundleProvider newList(IBaseResource theResource) { 079 return new SimpleBundleProvider(theResource); 080 } 081 082 public static IBundleProvider newList(List<IBaseResource> theResources) { 083 return new SimpleBundleProvider(theResources); 084 } 085}