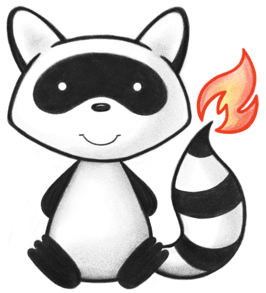
001/* 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.context.api.AddProfileTagEnum; 024import ca.uhn.fhir.interceptor.api.IInterceptorService; 025import ca.uhn.fhir.rest.api.EncodingEnum; 026import ca.uhn.fhir.rest.server.interceptor.IServerInterceptor; 027 028import java.util.List; 029 030public interface IRestfulServerDefaults { 031 /** 032 * @return Returns the setting for automatically adding profile tags 033 * @deprecated As of HAPI FHIR 1.5, this property has been moved to 034 * {@link FhirContext#setAddProfileTagWhenEncoding(AddProfileTagEnum)} 035 */ 036 @Deprecated 037 AddProfileTagEnum getAddProfileTag(); 038 039 /** 040 * @return Returns the default encoding to return (XML/JSON) if an incoming request does not specify a preference 041 * (either with the <code>_format</code> URL parameter, or with an <code>Accept</code> header 042 * in the request. The default is {@link EncodingEnum#XML}. Will not return null. 043 */ 044 EncodingEnum getDefaultResponseEncoding(); 045 046 /** 047 * @return Returns the server support for ETags (will not be <code>null</code>). Default is 048 * {@link RestfulServer#DEFAULT_ETAG_SUPPORT} 049 */ 050 ETagSupportEnum getETagSupport(); 051 052 /** 053 * @return Returns the support option for the <code>_elements</code> parameter on search 054 * and read operations. 055 * @see <a href="http://hapifhir.io/doc_rest_server.html#extended_elements_support">Extended Elements Support</a> 056 */ 057 ElementsSupportEnum getElementsSupport(); 058 059 /** 060 * Gets the {@link FhirContext} associated with this server. For efficient processing, resource providers and plain 061 * providers should generally use this context if one is needed, as opposed to 062 * creating their own. 063 */ 064 FhirContext getFhirContext(); 065 066 /** 067 * Returns the list of interceptors registered against this server 068 */ 069 List<IServerInterceptor> getInterceptors_(); 070 071 /** 072 * Returns the paging provider for this server 073 */ 074 IPagingProvider getPagingProvider(); 075 076 /** 077 * Default page size for searches. Null means no limit (JpaStorageSettings might have size limit however) 078 */ 079 default Integer getDefaultPageSize() { 080 return null; 081 } 082 083 /** 084 * Maximum page size for searches. Null means no upper limit. 085 */ 086 default Integer getMaximumPageSize() { 087 return null; 088 } 089 090 /** 091 * Should the server "pretty print" responses by default (requesting clients can always override this default by 092 * supplying an <code>Accept</code> header in the request, or a <code>_pretty</code> 093 * parameter in the request URL. 094 * <p> 095 * The default is <code>false</code> 096 * </p> 097 * 098 * @return Returns the default pretty print setting 099 */ 100 boolean isDefaultPrettyPrint(); 101 102 /** 103 * Returns the interceptor service for this server 104 */ 105 IInterceptorService getInterceptorService(); 106}