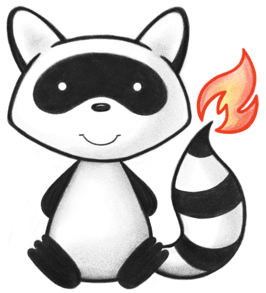
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.context.support.IValidationSupport; 024import ca.uhn.fhir.rest.api.server.RequestDetails; 025import ca.uhn.fhir.util.BundleUtil; 026import jakarta.annotation.Nonnull; 027import org.apache.commons.lang3.Validate; 028import org.hl7.fhir.instance.model.api.IBaseBundle; 029import org.hl7.fhir.instance.model.api.IBaseResource; 030 031import java.util.Collections; 032import java.util.List; 033 034public abstract class BaseResponseTerminologyInterceptor { 035 protected final IValidationSupport myValidationSupport; 036 protected final FhirContext myContext; 037 038 /** 039 * Constructor 040 * 041 * @param theValidationSupport The validation support module 042 */ 043 public BaseResponseTerminologyInterceptor(@Nonnull IValidationSupport theValidationSupport) { 044 myValidationSupport = theValidationSupport; 045 Validate.notNull(theValidationSupport, "The validation support must not be null"); 046 047 myContext = theValidationSupport.getFhirContext(); 048 Validate.notNull(myContext, "The validation support must not return a null context"); 049 } 050 051 @Nonnull 052 protected List<IBaseResource> toListForProcessing(RequestDetails theRequestDetails, IBaseResource theResource) { 053 054 switch (theRequestDetails.getRestOperationType()) { 055 // Don't apply to these operations 056 case ADD_TAGS: 057 case DELETE_TAGS: 058 case GET_TAGS: 059 case GET_PAGE: 060 case GRAPHQL_REQUEST: 061 case EXTENDED_OPERATION_SERVER: 062 case EXTENDED_OPERATION_TYPE: 063 case EXTENDED_OPERATION_INSTANCE: 064 case CREATE: 065 case DELETE: 066 case TRANSACTION: 067 case UPDATE: 068 case VALIDATE: 069 case METADATA: 070 case META_ADD: 071 case META: 072 case META_DELETE: 073 case PATCH: 074 default: 075 return Collections.emptyList(); 076 077 // Do apply to these operations 078 case HISTORY_INSTANCE: 079 case HISTORY_SYSTEM: 080 case HISTORY_TYPE: 081 case SEARCH_SYSTEM: 082 case SEARCH_TYPE: 083 case READ: 084 case VREAD: 085 break; 086 } 087 088 List<IBaseResource> resources; 089 if (theResource instanceof IBaseBundle) { 090 resources = BundleUtil.toListOfResources(myContext, (IBaseBundle) theResource); 091 } else { 092 resources = Collections.singletonList(theResource); 093 } 094 return resources; 095 } 096}