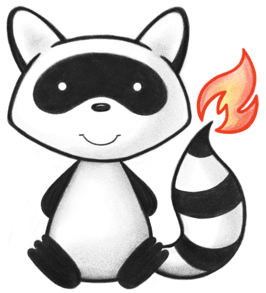
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.util.ClasspathUtil; 024import com.fasterxml.jackson.databind.ObjectMapper; 025import org.slf4j.Logger; 026import org.slf4j.LoggerFactory; 027 028import java.io.IOException; 029import java.io.StringReader; 030import java.util.Properties; 031 032public class ConfigLoader { 033 034 private static final Logger ourLog = LoggerFactory.getLogger(ConfigLoader.class); 035 public static final String CLASSPATH = "classpath:"; 036 037 public static String loadResourceContent(String theResourcePath) { 038 if (theResourcePath.startsWith(CLASSPATH)) { 039 theResourcePath = theResourcePath.substring(CLASSPATH.length()); 040 } 041 return ClasspathUtil.loadResource(theResourcePath); 042 } 043 044 public static Properties loadProperties(String theResourcePath) { 045 String propsString = loadResourceContent(theResourcePath); 046 Properties props = new Properties(); 047 try { 048 props.load(new StringReader(propsString)); 049 } catch (IOException e) { 050 throw new RuntimeException( 051 Msg.code(324) + String.format("Unable to load properties at %s", theResourcePath), e); 052 } 053 return props; 054 } 055 056 public static <T> T loadJson(String theResourcePath, Class<T> theModelClass) { 057 ObjectMapper mapper = new ObjectMapper(); 058 try { 059 return mapper.readValue(loadResourceContent(theResourcePath), theModelClass); 060 } catch (Exception e) { 061 throw new RuntimeException( 062 Msg.code(325) + String.format("Unable to parse resource at %s", theResourcePath), e); 063 } 064 } 065}